golang默認(rèn)的time.Time類型在轉(zhuǎn)為json格式時(shí)不是常用的2019-05-08 10:00:01這種格式,解決辦法是自定義一個(gè)時(shí)間類型,例如
type myTime time.Time ,然后針對(duì)myTime實(shí)現(xiàn)Marshaler接口的MarshalJSON方法,例如:
package models
import (
"database/sql/driver"
"time"
)
const localDateTimeFormat string = "2006-01-02 15:04:05"
type LocalTime time.Time
func (l LocalTime) MarshalJSON() ([]byte, error) {
b := make([]byte, 0, len(localDateTimeFormat)+2)
b = append(b, '"')
b = time.Time(l).AppendFormat(b, localDateTimeFormat)
b = append(b, '"')
return b, nil
}
func (l *LocalTime) UnmarshalJSON(b []byte) error {
now, err := time.ParseInLocation(`"`+localDateTimeFormat+`"`, string(b), time.Local)
*l = LocalTime(now)
return err
}
上面的代碼在網(wǎng)上隨手一搜就能找到,沒有什么困難的,接下來的才是本篇文章的重點(diǎn),這玩意結(jié)合xorm使用時(shí),特別是字段類型為*LocalTime的時(shí)候才需要折騰一番。
下面是我的對(duì)應(yīng)數(shù)據(jù)庫表結(jié)構(gòu)的struct 定義,
type ServerInfo struct {
ServerInfoId string `xorm:"varchar(32) pk server_info_id"`
CreatedAt LocalTime `xorm:"timestamp created"`
UpdatedAt LocalTime `xorm:"timestamp updated"`
DeletedAt *LocalTime `xorm:"timestamp deleted index"`
OrgId string `xorm:"varchar(100) org_id" json:"orgId"`
ServerIp string `xorm:"varchar(128) server_ip" json:"serverIp"`
ServerNameDesc string `xorm:"varchar(500) server_name_desc" json:"serverNameDesc"`
ServerTimeNow LocalTime `xorm:"timestamp server_time" json:"serverTime"`
DataReceiveTime LocalTime `xorm:"timestamp data_receive_time" sql:"DEFAULT:current_timestamp" json:"dataRecvTime"`
LastUploadDataTime *LocalTime `xorm:"timestamp last_upload_data_time" json:"lastUploadDataTime"`
LastCheckTime *LocalTime `xorm:"timestamp last_check_time" json:"lastCheckTime"`
LastErrorTime *LocalTime `xorm:"timestamp last_error_time" json:"lastErrorTime"`
}
注意上面的字段類型,既有LocalTime類型的,又有*LocalTime類型的,*LocalTime是考慮到有時(shí)候數(shù)據(jù)值可能為NULL,即字段值可能為空的情況。
xorm不知道如何為LocalTime這個(gè)自定義類型進(jìn)行賦值或者取值,因此需要實(shí)現(xiàn)xorm的core包中的Conversion接口,這個(gè)接口的定義如下:

注意,坑已經(jīng)隱藏在上面的接口定義中了,過一會(huì)說。
整個(gè)完整的自定義時(shí)間類型的代碼變成了下面的這樣:
package models
import (
"database/sql/driver"
"time"
)
const localDateTimeFormat string = "2006-01-02 15:04:05"
type LocalTime time.Time
func (l LocalTime) MarshalJSON() ([]byte, error) {
b := make([]byte, 0, len(localDateTimeFormat)+2)
b = append(b, '"')
b = time.Time(l).AppendFormat(b, localDateTimeFormat)
b = append(b, '"')
return b, nil
}
func (l *LocalTime) UnmarshalJSON(b []byte) error {
now, err := time.ParseInLocation(`"`+localDateTimeFormat+`"`, string(b), time.Local)
*l = LocalTime(now)
return err
}
func (l LocalTime) String() string {
return time.Time(l).Format(localDateTimeFormat)
}
func (l LocalTime)Now()(LocalTime){
return LocalTime(time.Now())
}
func (l LocalTime)ParseTime(t time.Time)(LocalTime){
return LocalTime(t)
}
func (j LocalTime) format() string {
return time.Time(j).Format(localDateTimeFormat)
}
func (j LocalTime) MarshalText() ([]byte, error) {
return []byte(j.format()), nil
}
func (l *LocalTime) FromDB(b []byte) error {
if nil == b || len(b) == 0 {
l = nil
return nil
}
var now time.Time
var err error
now, err = time.ParseInLocation(localDateTimeFormat, string(b), time.Local)
if nil == err {
*l = LocalTime(now)
return nil
}
now, err = time.ParseInLocation("2006-01-02T15:04:05Z", string(b), time.Local)
if nil == err {
*l = LocalTime(now)
return nil
}
panic("自己定義個(gè)layout日期格式處理一下數(shù)據(jù)庫里面的日期型數(shù)據(jù)解析!")
return err
}
//func (t *LocalTime) Scan(v interface{}) error {
// // Should be more strictly to check this type.
// vt, err := time.Parse("2006-01-02 15:04:05", string(v.([]byte)))
// if err != nil {
// return err
// }
// *t = LocalTime(vt)
// return nil
//}
func (l *LocalTime) ToDB() ([]byte, error) {
if nil == l {
return nil,nil
}
return []byte(time.Time(*l).Format(localDateTimeFormat)), nil
}
func (l *LocalTime) Value() (driver.Value, error) {
if nil==l {
return nil, nil
}
return time.Time(*l).Format(localDateTimeFormat), nil
}
此時(shí),要是數(shù)據(jù)庫的字段內(nèi)容都有值的話插入和更新應(yīng)該是沒有什么問題,但是*LocalTime字段的值為nil的話問題就開始出現(xiàn)了,上面說了,ToDB()方法的返回值類型為[]byte,當(dāng)字段值為nil時(shí),返回nil看上去一切正常,但是xorm打印出來的sql語句數(shù)據(jù)值是下面這個(gè)樣子的:

這個(gè)[]uint8(nil)就是*LocalTime值為nil時(shí)的情況,數(shù)據(jù)庫驅(qū)動(dòng)是不認(rèn)可[]uint8(nil)這種數(shù)據(jù)去寫給timestamp類型字段的,問題的根源就是ToDB方法的返回值類型為[]byte,既然是這樣,就需要我們?nèi)藶榈陌裑]uint8(nil)這種類型改為interface(nil)類型,數(shù)據(jù)庫驅(qū)動(dòng)會(huì)識(shí)別interface(nil)為NULL值,修改代碼xorm\statement.go第322行,把原來的val=data改成下面的樣子:
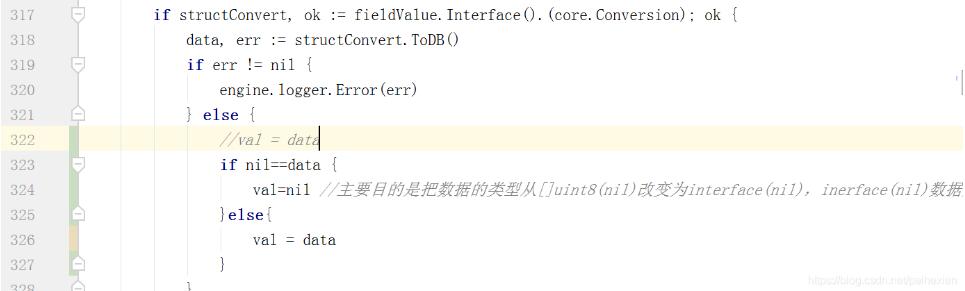
就是把val=data改為 if nil==data { val=nil } else {val=data} ,看上去邏輯沒有什么變化,但是給val=nil賦值的時(shí)候,val的類型就從[]uint8(nil)變成了interface(nil)了,這樣數(shù)據(jù)庫驅(qū)動(dòng)就可以正確處理空值了。
除了需要修改xorm\statement.go文件的內(nèi)容,還需要修改xorm\session_convert.go的第558行,增加以下代碼:
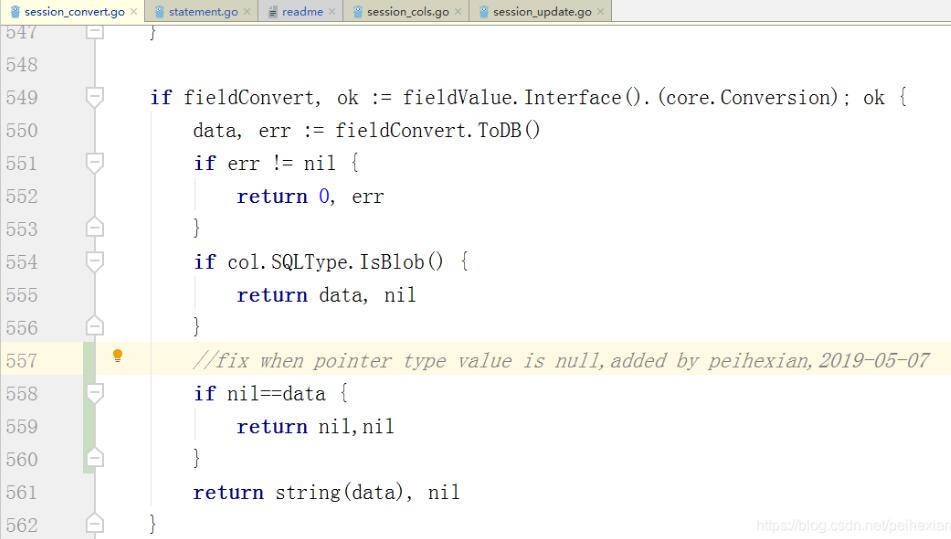
主要是增加下面的代碼
//fix when pointer type value is null,added by peihexian,2019-05-07
if nil==data {
return nil,nil
}
之所以加這個(gè)代碼是因?yàn)閤orm作者沒有考慮指針類型字段值為nil的情況,xorm對(duì)有轉(zhuǎn)換的字段要么當(dāng)成數(shù)字,要么當(dāng)成了字符串,這兩種對(duì)于NULL類型的值都不適用,所以需要增加if nil==data return nil,nil這樣的代碼,還是把數(shù)據(jù)值組織成interface(nil)去給數(shù)據(jù)庫驅(qū)動(dòng)去處理。
另外還有一個(gè)地方,是session_convert.go 第556行,同樣需要增加
if nil==data { //edit by peihexian 2019.06.19
return nil,nil
}
下面是加完以后的樣子
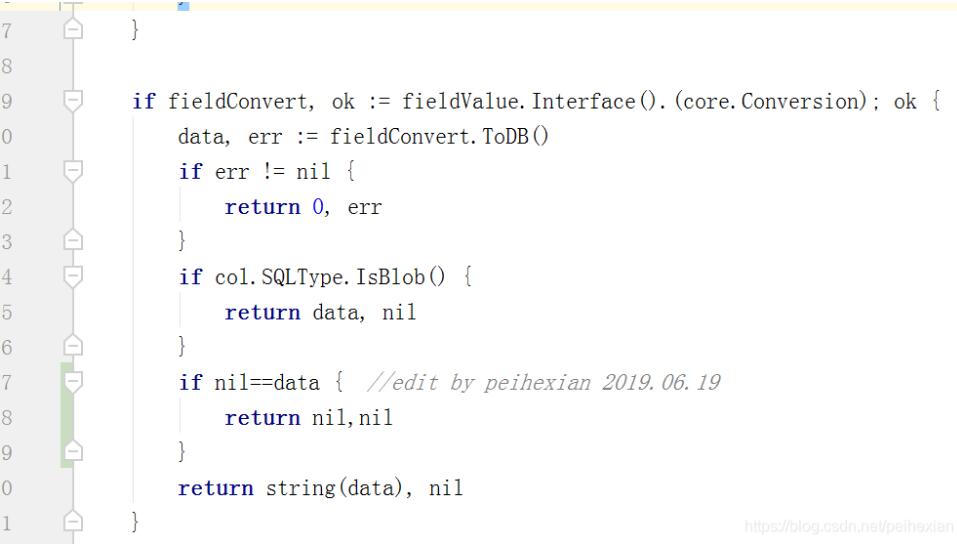
到這里,對(duì)xorm做了幾處小的修改,自定義日期的問題及json格式化問題完美解決。
以上為個(gè)人經(jīng)驗(yàn),希望能給大家一個(gè)參考,也希望大家多多支持腳本之家。如有錯(cuò)誤或未考慮完全的地方,望不吝賜教。
您可能感興趣的文章:- golang 定時(shí)任務(wù)方面time.Sleep和time.Tick的優(yōu)劣對(duì)比分析
- 解決Golang time.Parse和time.Format的時(shí)區(qū)問題
- 解決golang時(shí)間字符串轉(zhuǎn)time.Time的坑
- golang的時(shí)區(qū)和神奇的time.Parse的使用方法
- 對(duì)Golang中的runtime.Caller使用說明
- Golang中的time.Duration類型用法說明
- golang time包做時(shí)間轉(zhuǎn)換操作
- golang time常用方法詳解