注冊功能實現(xiàn)
- forms組件進行表單驗證;
- 用戶頭像前端實時展示;
- ajax發(fā)送post請求;
應(yīng)用forms組件實現(xiàn)用戶輸入信息的校驗。首先在app目錄下創(chuàng)建一個myform.py的文件。
如果你的項目至始至終只用到一個forms組件那么你可以直接建一個py文件書寫即可。
但是如果你的項目需要使用多個forms組件,那么你可以創(chuàng)建一個myforms文件夾在文件夾內(nèi),根據(jù)forms組件功能的不同創(chuàng)建不同的py文件。
- regform.py
- loginform.py
- userform.py
- orderform.py
......
# 書寫針對用戶表的forms主鍵代碼
from django import forms
from app01 import models
class MyRegForm(forms.Form):
username = forms.CharField(label='用戶名',min_length=3,max_length=8,
error_messages={
'required':'用戶名不能為空',
'min_length':'用戶名最少3位',
'max_length':'用戶名最大8位'
},
# 還需要讓標(biāo)簽有Bootstrap樣式
widget=forms.widgets.TextInput(attrs={'class':'form-control'})
)
password = forms.CharField(label='密碼',min_length=3,max_length=8,
error_messages={
'required':'密碼不能為空',
'min_length':'密碼最少3位',
'max_length':'密碼最大8位'
},
# 還需要讓標(biāo)簽有Bootstrap樣式
widget=forms.widgets.PasswordInput(attrs={'class':'form-control'})
)
confirm_password = forms.CharField(label='確認密碼',min_length=3,max_length=8,
error_messages={
'required':'確認密碼不能為空',
'min_length':'確認密碼最少3位',
'max_length':'確認密碼最大8位'
},
# 還需要讓標(biāo)簽有Bootstrap樣式
widget=forms.widgets.PasswordInput(attrs={'class':'form-control'})
)
email = forms.EmailField(label='郵箱',
error_messages={
'required': '郵箱不能為空',
'invalid':'郵箱格式不正確',
},
widget=forms.widgets.EmailInput(attrs={'class':'form-control'})
)
# 鉤子函數(shù)
# 局部鉤子:校驗用戶名是否已存在
def clean_username(self):
username = self.cleaned_data.get('username')
# 去數(shù)據(jù)庫中校驗
is_exist = models.UserInfo.objects.filter(username=username)
if is_exist:
# 提示信息
self.add_error('username','用戶名已存在')
return username
# 全局鉤子:校驗兩次密碼是否一致
def clean(self):
password = self.cleaned_data.get('password')
confirm_password = self.cleaned_data.get('confirm_password')
if not password == confirm_password:
self.add_error('confirm_password','兩次密碼不一致')
return self.cleaned_data
然后在urls.py
中配置注冊頁的路由信息。
from django.contrib import admin
from django.urls import path
from app01 import views
urlpatterns = [
path('admin/', admin.site.urls),
path('register/',views.register,name='reg'),
]
在視圖函數(shù)views.py
中編寫forms組件檢驗、ajax發(fā)送的post請求獲取數(shù)據(jù)、調(diào)用django orm功能存儲數(shù)據(jù)、將后端的處理結(jié)果返回給前端進行校驗。
from app01.myforms import MyRegForm
from app01 import models
from django.http import JsonResponse
# Create your views here.
def register(request):
form_obj = MyRegForm()
if request.method == 'POST':
# 定義返回給前端的js數(shù)據(jù)結(jié)果
back_dic = {"code": 1000, 'msg': ''}
# 校驗數(shù)據(jù)是否合法
form_obj = MyRegForm(request.POST)
# 判斷數(shù)據(jù)是否合法
if form_obj.is_valid():
# form_obj.cleaned_data:{'username': 'zhangsan', 'password': '123456', 'confirm_password': '123456', 'email': '123@qq.com'}
# 將校驗通過的數(shù)據(jù)字典賦值給一個變量
clean_data = form_obj.cleaned_data
# 將字典里面的confirm_password鍵值對刪除
clean_data.pop('confirm_password') # {'username': 'zhangsan', 'password': '123456', 'email': '123@qq.com'}
# 注意用戶頭像是一個圖片的文件,request.POST中只有鍵值對的數(shù)據(jù)
file_obj = request.FILES.get('avatar')
"""
針對用戶頭像一定要判斷是否傳值,不能直接添加到字典里面去
否則file_obj=None,會將數(shù)據(jù)庫中默認的圖片路徑覆蓋。
"""
if file_obj:
# 向字典數(shù)據(jù)clean_data中增加一個圖片頭像的字段
clean_data['avatar'] = file_obj
# 操作數(shù)據(jù)庫保存數(shù)據(jù)
models.UserInfo.objects.create_user(**clean_data)
# 注冊成功則跳轉(zhuǎn)到登錄頁面
back_dic['url'] = '/login/'
else:
back_dic['code'] = 2000 # 校驗存在錯誤
back_dic['msg'] = form_obj.errors
# 將字典類型的數(shù)據(jù)封裝成json返回到前端
return JsonResponse(back_dic)
return render(request,'register.html',locals())
前端的注冊頁面:register.html
!DOCTYPE html>
html lang="en">
head>
meta charset="UTF-8">
!-- Bootstrap -->
link rel="external nofollow" rel="stylesheet">
script src="https://cdn.jsdelivr.net/npm/jquery@1.12.4/dist/jquery.min.js">
script src="https://cdn.jsdelivr.net/npm/bootstrap@3.3.7/dist/js/bootstrap.min.js">/script>
title>用戶注冊/title>
/head>
body>
div class="container-fluid">
div class="row">
div class="col-md-8 col-md-offset-2">
h1 class="text-center">注冊/h1>
form id="myform"> !--這里我們不用form表單提交數(shù)據(jù) 知識單純的用一下form標(biāo)簽而已-->
{% csrf_token %}
{% for form in form_obj %}
div class="form-group">
label for="{{ form.auto_id }}">{{ form.label }}/label>
{{ form }}
span style="color: red" class="pull-right">/span>
/div>
{% endfor %}
div class="form-group">
label for="myfile">頭像
{% load static %}
img src="{% static 'img/default.jpg' %}" id='myimg' alt="" width="100" style="margin-left: 10px">
/label>
input type="file" id="myfile" name="avatar" style="display: none" >
/div>
input type="button" class="btn btn-primary pull-right" value="注冊" id="id_commit">
/form>
/div>
/div>
/div>
/body>
/html>
【重難點】在于書寫JS處理邏輯:包括了圖片上傳加載、ajax發(fā)送的post請求以及后端注冊結(jié)果的信息處理。
script>
$("#myfile").change(function () {
// 文件閱讀器對象
// 1 先生成一個文件閱讀器對象
let myFileReaderObj = new FileReader();
// 2 獲取用戶上傳的頭像文件
let fileObj = $(this)[0].files[0];
// 3 將文件對象交給閱讀器對象讀取
myFileReaderObj.readAsDataURL(fileObj) // 異步操作 IO操作
// 4 利用文件閱讀器將文件展示到前端頁面 修改src屬性
// 等待文件閱讀器加載完畢之后再執(zhí)行
myFileReaderObj.onload = function(){
$('#myimg').attr('src',myFileReaderObj.result)
}
})
$('#id_commit').click(function () {
// 發(fā)送ajax請求 我們發(fā)送的數(shù)據(jù)中即包含普通的鍵值也包含文件
let formDataObj = new FormData();
// 1.添加普通的鍵值對
{#console.log($('#myform').serializeArray()) // [{},{},{},{},{}] 只包含普通鍵值對#}
$.each($('#myform').serializeArray(),function (index,obj) {
{#console.log(index,obj)#} // obj = {}
formDataObj.append(obj.name,obj.value)
});
// 2.添加文件數(shù)據(jù)
formDataObj.append('avatar',$('#myfile')[0].files[0]);
// 3.發(fā)送ajax請求
$.ajax({
url:"",
type:'post',
data:formDataObj,
// 需要指定兩個關(guān)鍵性的參數(shù)
contentType:false,
processData:false,
success:function (args) {
if (args.code==1000){
// 跳轉(zhuǎn)到登陸頁面
//window.location.href = args.url
}else{
// 如何將對應(yīng)的錯誤提示展示到對應(yīng)的input框下面
// forms組件渲染的標(biāo)簽的id值都是 id_字段名
$.each(args.msg,function (index,obj) {
{#console.log(index,obj) // username ["用戶名不能為空"]#}
let targetId = '#id_' + index;
$(targetId).next().text(obj[0]).parent().addClass('has-error')
})
}
}
})
})
// 給所有的input框綁定獲取焦點事件
$('input').focus(function () {
// 將input下面的span標(biāo)簽和input外面的div標(biāo)簽修改內(nèi)容及屬性
$(this).next().text('').parent().removeClass('has-error')
})
/script>
效果如下:
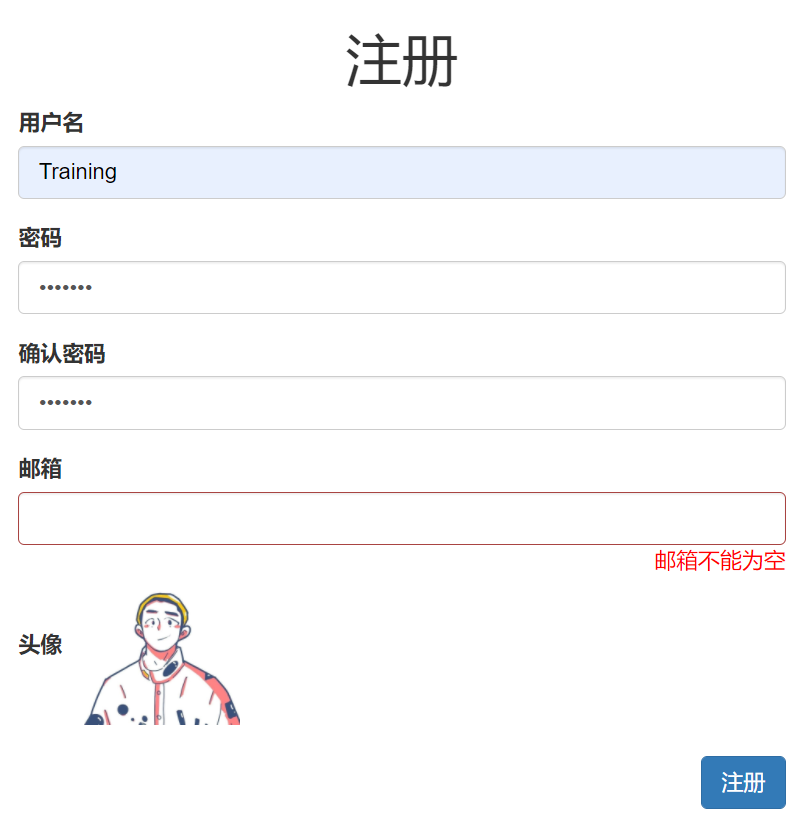
以上就是通用的Django注冊功能模塊實現(xiàn)步驟的詳細內(nèi)容,更多關(guān)于Django注冊功能模塊實現(xiàn)的資料請關(guān)注腳本之家其它相關(guān)文章!
您可能感興趣的文章:- django+vue實現(xiàn)注冊登錄的示例代碼
- django注冊用郵箱發(fā)送驗證碼的實現(xiàn)
- Django怎么在admin后臺注冊數(shù)據(jù)庫表
- Django用戶登錄與注冊系統(tǒng)的實現(xiàn)示例
- django 框架實現(xiàn)的用戶注冊、登錄、退出功能示例
- django實現(xiàn)用戶注冊實例講解
- Django實現(xiàn)auth模塊下的登錄注冊與注銷功能
- Python Django 實現(xiàn)簡單注冊功能過程詳解
- django的登錄注冊系統(tǒng)的示例代碼
- django 通過ajax完成郵箱用戶注冊、激活賬號的方法
- Django商城項目注冊功能的實現(xiàn)