在日常工作中,Python在辦公自動(dòng)化領(lǐng)域應(yīng)用非常廣泛,如批量將多個(gè)Excel中的數(shù)據(jù)進(jìn)行計(jì)算并生成圖表,批量將多個(gè)Excel按固定格式轉(zhuǎn)換成Word,或者定時(shí)生成文件并發(fā)送郵件等場(chǎng)景。本文主要以一個(gè)簡(jiǎn)單的小例子,簡(jiǎn)述Python在Excel和Word方面進(jìn)行相互轉(zhuǎn)換的相關(guān)知識(shí)點(diǎn),謹(jǐn)供學(xué)習(xí)分享使用,如有不足之處,還請(qǐng)指正。
相關(guān)知識(shí)點(diǎn)
本文主要是將Excel文件通過(guò)一定規(guī)則轉(zhuǎn)換成Word文檔,涉及知識(shí)點(diǎn)如下所示:
xlrd模塊:主要用于Excel文件的讀取,相關(guān)內(nèi)容如下:
- xlrd.open_workbook(self.excel_file) 打開(kāi)Excel文件并返回文檔對(duì)象,參數(shù)為Excel的完整路徑
- book.sheet_by_name(self.sheet_name) 通過(guò)名稱獲取對(duì)應(yīng)的sheet頁(yè),并返回sheet對(duì)象
- sheet.nrows sheet頁(yè)的有效行數(shù)
- sheet.ncols sheet頁(yè)的有效列數(shù)
- sheet.row_values(0) 返回Excel中對(duì)應(yīng)sheet頁(yè)的第一行的值,以數(shù)組返回
- sheet.cell_value(row, col) 返回某一個(gè)單元格的值
python-docx模塊:主要操作Word文檔,如:表格,段落等相關(guān),相關(guān)內(nèi)容如下所示:
- Document word的文檔對(duì)象,代表整個(gè)word文檔
- doc.sections[0] 獲取章節(jié)
- doc.add_section(start_type=WD_SECTION_START.CONTINUOUS) 添加連續(xù)章節(jié)
- doc.add_heading(third, level=2) 增加標(biāo)題,level表示級(jí)別,如二級(jí)標(biāo)題,返回標(biāo)題對(duì)象
- doc.add_paragraph(text='', style=None) 增加段落,返回段落對(duì)象
- doc.add_table(rows=4, cols=5) 增加表格,并返回表格對(duì)象
- doc_table.style = "Table Grid" 設(shè)置表格樣式
- doc_table.rows[0].cells[1].merge(doc_table.rows[0].cells[4]) 合并單元格
- doc_table.rows[3].cells 獲取表格某一行所有單元格,以數(shù)組形式返回
- head_cells[0].width = Cm(1.9) 設(shè)置列寬,單位cm
- doc_table.rows[i].cells[j].vertical_alignment = WD_CELL_VERTICAL_ALIGNMENT.CENTER 表格內(nèi)容垂直居中
- doc_table.add_row() 新增行,并返回行對(duì)象
插件安裝
插件可以在pycharm的terminal面板下進(jìn)行安裝。python-docx安裝命令為:pip install python-docx
xlrd安裝命令為:pip install xlrd
如下所示:
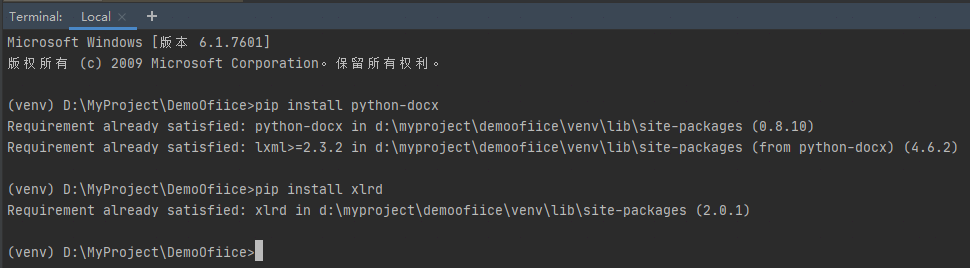
數(shù)據(jù)源文件
數(shù)據(jù)源是一系列格式相同的Excel文件,共七列,其中第1列要按【/】進(jìn)行截取拆分,格式如下:

核心代碼
本文核心源碼,主要分三部分:
導(dǎo)入相關(guān)模塊包,如下所示:
import xlrd
from docx import Document
from docx.enum.section import WD_ORIENTATION
from docx.enum.text import WD_PARAGRAPH_ALIGNMENT
from docx.shared import Pt, Cm, RGBColor
from docx.oxml.ns import qn
from docx.enum.table import WD_CELL_VERTICAL_ALIGNMENT
讀取Excel,如下所示:
def read_excel(self):
"""讀取Excel"""
book = xlrd.open_workbook(self.excel_file)
sheet = book.sheet_by_name(self.sheet_name)
nrows = sheet.nrows # 行數(shù)
ncols = sheet.ncols # 列數(shù)
datas = [] # 存放數(shù)據(jù)
# 第一列 標(biāo)題
keys = sheet.row_values(0)
for row in range(1, nrows):
data = {} # 每一行數(shù)據(jù)
for col in range(0, ncols):
value = sheet.cell_value(row, col) # 取出每一個(gè)單元格的數(shù)據(jù)
# 替換到特殊字符
value = value.replace('', '').replace('>', '').replace('$', '')
data[keys[col]] = value
# 截取第一列元素
if col == 0:
first = '' # 截取元素 第1
second = '' # 截取元素 第2
third = '' # 截取元素 第3
arrs = value.lstrip('/').split('/') # 去掉第一個(gè)/ 然后再以/分組
if len(arrs) > 0:
if len(arrs) == 1:
first = arrs[0]
second = first
third = second
elif len(arrs) == 2:
first = arrs[0]
second = arrs[1]
third = second
elif len(arrs) == 3:
first = arrs[0]
second = arrs[1]
third = arrs[2]
else:
first = arrs[0]
second = arrs[1]
third = arrs[2]
else:
first = value.ltrip('/')
second = first
third = second
data['first'] = first
data['second'] = second
data['third'] = third
# 截取第一列結(jié)束
datas.append(data)
return datas
生成Word部分:
def write_word(self, datas):
"""生成word文件"""
if len(datas) 1:
print('Excel沒(méi)有內(nèi)容')
return
# 定義word文檔對(duì)象
doc = Document()
# 添加橫向
section = doc.sections[0] # doc.add_section(start_type=WD_SECTION_START.CONTINUOUS) # 添加橫向頁(yè)的連續(xù)節(jié)
section.orientation = WD_ORIENTATION.LANDSCAPE
page_h, page_w = section.page_width, section.page_height
section.page_width = page_w # 設(shè)置橫向紙的寬度
section.page_height = page_h # 設(shè)置橫向紙的高度
# 設(shè)置字體
doc.styles['Normal'].font.name = u'宋體'
doc.styles['Normal']._element.rPr.rFonts.set(qn('w:eastAsia'), u'宋體')
# 獲取第3部分(部門) 并去重
data_third = []
for data in datas:
third = data['third']
if data_third.count(third) == 0:
data_third.append(third)
for third in data_third:
h2 = doc.add_heading(third, level=2) # 寫(xiě)入部門,二級(jí)標(biāo)題
run = h2.runs[0] # 可以通過(guò)add_run來(lái)設(shè)置文字,也可以通過(guò)數(shù)組來(lái)獲取
run.font.color.rgb = RGBColor(0, 0, 0)
run.font.name = u'宋體'
doc.add_paragraph(text='', style=None) # 增加空白行 換行
# 開(kāi)始獲取模板
data_template = []
for data in datas:
if data['third'] == third:
template = {'first': data['first'], '模板名稱': data['模板名稱']}
if data_template.count(template) == 0:
data_template.append(template)
# 獲取模板完成
# 遍歷模板
for template in data_template:
h3 = doc.add_heading(template['模板名稱'], level=3) # 插入模板名稱,三級(jí)標(biāo)題
run = h3.runs[0] # 可以通過(guò)add_run來(lái)設(shè)置文字,也可以通過(guò)數(shù)組來(lái)獲取
run.font.color.rgb = RGBColor(0, 0, 0)
run.font.name = u'宋體'
doc.add_paragraph(text='', style=None) # 換行
data_table = filter(
lambda data: data['third'] == third and data['模板名稱'] == template['模板名稱'] and data['first'] ==
template['first'], datas)
data_table = list(data_table)
# 新增表格 4行5列
doc_table = doc.add_table(rows=4, cols=5)
doc_table.style = "Table Grid"
doc_table.style.font.size = Pt(9)
doc_table.style.font.name = '宋體'
# 合并單元格 賦值
doc_table.rows[0].cells[1].merge(doc_table.rows[0].cells[4])
doc_table.rows[1].cells[1].merge(doc_table.rows[1].cells[4])
doc_table.rows[2].cells[1].merge(doc_table.rows[2].cells[4])
doc_table.rows[0].cells[0].text = '流程名稱:'
doc_table.rows[0].cells[1].text = data_table[0]['模板名稱']
doc_table.rows[1].cells[0].text = '使用人:'
doc_table.rows[1].cells[1].text = data_table[0]['first']
doc_table.rows[2].cells[0].text = '流程說(shuō)明:'
doc_table.rows[2].cells[1].text = data_table[0]['流程說(shuō)明']
# 設(shè)置標(biāo)題
head_cells = doc_table.rows[3].cells # 前面還有三行,特殊處理
head_cells[0].text = '節(jié)點(diǎn)'
head_cells[1].text = '節(jié)點(diǎn)名'
head_cells[2].text = '處理人員'
head_cells[3].text = '處理方式'
head_cells[4].text = '跳轉(zhuǎn)信息'
# 設(shè)置列寬
head_cells[0].width = Cm(1.9)
head_cells[1].width = Cm(4.83)
head_cells[2].width = Cm(8.25)
head_cells[3].width = Cm(2.54)
head_cells[4].width = Cm(5.64)
# 第1 列水平居中,并設(shè)置行高,所有單元格內(nèi)容垂直居中
for i in range(0, 4):
# 水平居中
p = doc_table.rows[i].cells[0].paragraphs[0]
p.alignment = WD_PARAGRAPH_ALIGNMENT.CENTER
doc_table.rows[i].height = Cm(0.6) # 行高
# 垂直居中
for j in range(0, 5):
doc_table.rows[i].cells[j].vertical_alignment = WD_CELL_VERTICAL_ALIGNMENT.CENTER
# 生成表格并填充內(nèi)容
row_num = 0
for data in data_table:
row = doc_table.add_row()
row_cells = row.cells
row_cells[0].text = str(row_num + 1) # 序號(hào),需要轉(zhuǎn)換成字符串
row_cells[1].text = data['節(jié)點(diǎn)名稱']
row_cells[2].text = data['審批人員']
row_cells[3].text = data['審批方式']
row_cells[4].text = ''
# 水平居中
p = row_cells[0].paragraphs[0]
p.alignment = WD_PARAGRAPH_ALIGNMENT.CENTER
row.height = Cm(0.6) # 行高
# 垂直居中
for j in range(0, 5):
row_cells[j].vertical_alignment = WD_CELL_VERTICAL_ALIGNMENT.CENTER
row_num = row_num + 1
doc.add_paragraph(text='', style=None) # 換行
doc.save(self.word_file)
以上就是python 將Excel轉(zhuǎn)Word的示例的詳細(xì)內(nèi)容,更多關(guān)于python Excel轉(zhuǎn)Word的資料請(qǐng)關(guān)注腳本之家其它相關(guān)文章!
您可能感興趣的文章:- python提取word文件中的所有圖片
- python實(shí)現(xiàn)某考試系統(tǒng)生成word試卷
- 使用Python自動(dòng)化Microsoft Excel和Word的操作方法
- Python 制作詞云的WordCloud參數(shù)用法說(shuō)明
- Python WordCloud 修改色調(diào)的實(shí)現(xiàn)方式
- Python 自動(dòng)化修改word的案例
- Python實(shí)現(xiàn)Word文檔轉(zhuǎn)換Markdown的示例
- python3處理word文檔實(shí)例分析
- Python word文本自動(dòng)化操作實(shí)現(xiàn)方法解析
- Python快速優(yōu)雅的批量修改Word文檔樣式