昨天寫程序遇到一個(gè)問(wèn)題,pyqt5 加載常規(guī)的圖片完全可以顯示??僧?dāng)加載超清的高分辨率圖片時(shí),只能顯示一個(gè)小角落。可我就想把一張 3840x2160 的圖片加載到一個(gè) 800x600 的標(biāo)簽里該怎么辦呢?如何自適應(yīng)放縮尺寸,國(guó)內(nèi)社區(qū)眾所周知大多是抄襲,沒(méi)什么解決方案;外網(wǎng)站搜了一下也沒(méi)找到現(xiàn)成的解決方案,我知道又到了我開坑的時(shí)候了。
常規(guī)加載
先來(lái)看一下,如何借助 QLabel 和 QFileDialog 加載低分辨率的圖片,這時(shí)候時(shí)能正常顯示的。
import sys
from PyQt5.QtWidgets import (QMainWindow, QWidget, QHBoxLayout, QApplication,
QPushButton, QLabel, QFileDialog, QVBoxLayout,
QLineEdit)
from PyQt5.QtGui import QPixmap
class mainwindow(QMainWindow):
def __init__(self):
super(mainwindow, self).__init__()
layout = QVBoxLayout()
w = QWidget()
w.setLayout(layout)
self.setCentralWidget(w)
self.image_label = QLabel()
self.image_label.setFixedSize(800, 500)
layout.addWidget(self.image_label)
tmp_layout = QHBoxLayout()
btn = QPushButton("選擇圖片路徑")
tmp_layout.addWidget(btn)
btn.clicked.connect(self.load_image)
self.result = QLineEdit()
self.result.setPlaceholderText("車牌展示")
self.result.setReadOnly(True)
tmp_layout.addWidget(self.result)
layout.addLayout(tmp_layout)
def load_image(self):
fname, _ = QFileDialog.getOpenFileName(self, 'Open File',
'C://', "Image files (*.jpg *.png)")
if fname is not None:
pixmap = QPixmap(fname)
self.image_label.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication([])
m = mainwindow()
m.show()
sys.exit(app.exec())
上述代碼中,點(diǎn)擊『選擇圖片路徑』按鈕就會(huì)調(diào)用文件對(duì)話框,選擇圖片后就會(huì)打開。步驟為:
- 第一步,QFileDialog 選擇文件路徑
- 第二步,將文件路徑傳入 QPixmap 類,通過(guò)重載構(gòu)造一個(gè)對(duì)象,文檔原話:Constructs a pixmap from the file with the given fileName. If the file does not exist or is of an unknown format, the pixmap becomes a null pixmap.
- 第三步,將 QPixmap 對(duì)象傳給標(biāo)簽的 setPixmap 方法,就完成了圖片的顯示。
對(duì)于低分辨率圖片,加載是沒(méi)問(wèn)題的:
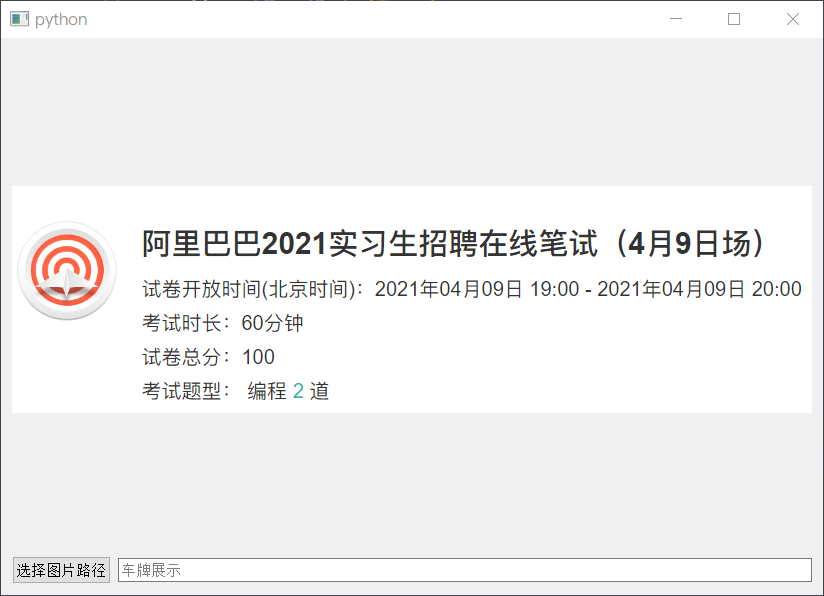
但高分辨率的圖片,只能顯示一個(gè)角落,也就是藍(lán)色框那一部分:
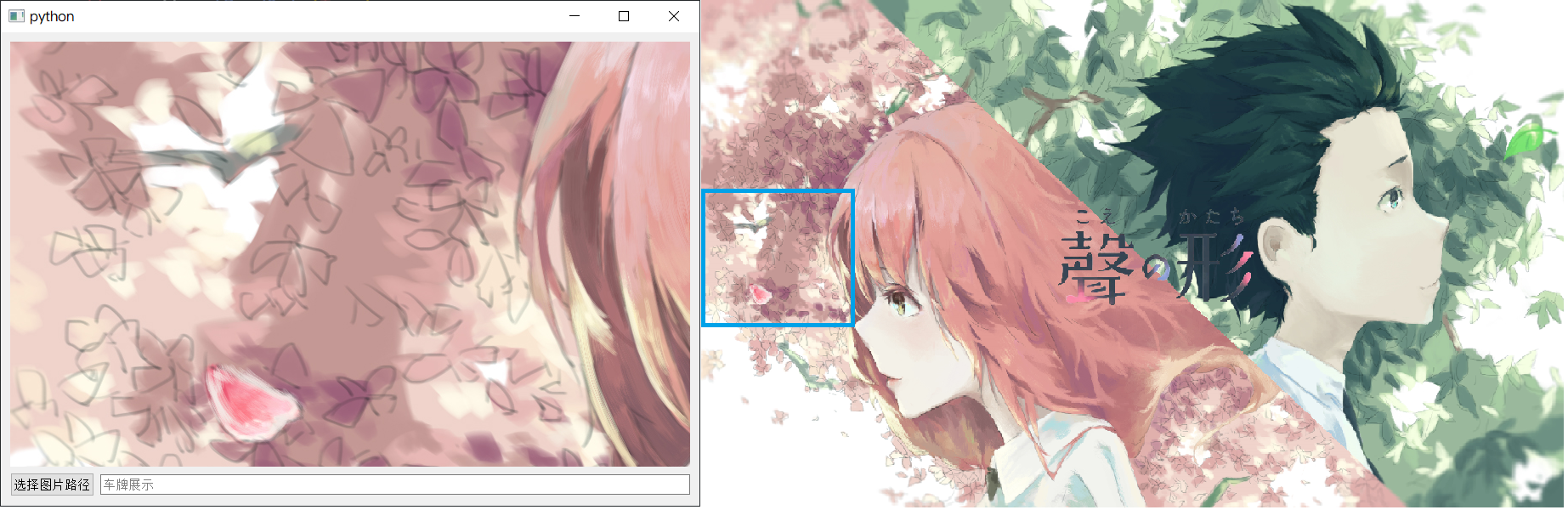
如何解決呢?既然國(guó)內(nèi)外都沒(méi)有現(xiàn)成的解決方案,只能掏出萬(wàn)能的官方文檔了。
QImageReader 類
需要注意的是官方文檔的語(yǔ)言是 C++,還好我會(huì)C++。打開文檔,映入眼簾的就四句話:
- QImageReader reader("large.jpeg"); 讀取圖片
- reader.size(); 圖片尺寸
- reader.setClipRect(myRect); 圖片裁剪
- reader.setScaledSize(mySize); 設(shè)置圖片尺寸,文檔原話:Another common function is to show a smaller version of the image. Loading a very large image and then scaling it down to the approriate size can be a very memory consuming operation. By calling the QImageReader::setScaledSize function, you can set the size that you want your resulting image to be.
剩下的任務(wù)就很簡(jiǎn)單了,讀圖片,設(shè)置尺寸,顯示。
import sys, time
from PyQt5.QtWidgets import (QMainWindow, QWidget, QHBoxLayout, QApplication,
QPushButton, QLabel, QFileDialog, QVBoxLayout,
QLineEdit)
from PyQt5.QtGui import QPixmap, QFont
from PyQt5.Qt import QSize, QImageReader
import qdarkstyle
class mainwindow(QMainWindow):
def __init__(self):
super(mainwindow, self).__init__()
layout = QVBoxLayout()
w = QWidget()
w.setLayout(layout)
self.setCentralWidget(w)
self.image_label = QLabel()
self.image_label.setFixedSize(800, 500)
layout.addWidget(self.image_label)
tmp_layout = QHBoxLayout()
btn = QPushButton("選擇圖片路徑")
tmp_layout.addWidget(btn)
btn.clicked.connect(self.load_image)
self.result = QLineEdit()
self.result.setPlaceholderText("車牌展示")
self.result.setReadOnly(True)
tmp_layout.addWidget(self.result)
layout.addLayout(tmp_layout)
self.setStyleSheet(qdarkstyle.load_stylesheet_pyqt5())
def load_image(self):
fname, _ = QFileDialog.getOpenFileName(self, 'Open File',
'C://', "Image files (*.jpg *.png)")
if fname is not None:
# 還需要對(duì)圖片進(jìn)行重新調(diào)整大小
img = QImageReader(fname)
scale = 800 / img.size().width()
height = int(img.size().height() * scale)
img.setScaledSize(QSize(800, height))
img = img.read()
# 打開設(shè)置好的圖片
pixmap = QPixmap(img)
self.image_label.setPixmap(pixmap)
self.result.setText("車牌號(hào)放到這里")
if __name__ == '__main__':
app = QApplication([])
font = QFont()
font.setFamily("SimHei")
font.setPointSize(14)
app.setFont(font)
m = mainwindow()
m.show()
sys.exit(app.exec())
考慮到可能會(huì)加載超清圖像,為了方便對(duì)圖片進(jìn)行控制,不要采用 QImage 或 QPixmap,而是使用 QImageReader
代碼解析:
- 創(chuàng)建 QImageReader 對(duì)象,方便對(duì)圖片進(jìn)行更多的操作
- 自適應(yīng)伸縮,將寬度限定為 800,自適應(yīng)計(jì)算高度應(yīng)該是多少,而后設(shè)置要縮放的大小
- 將設(shè)置好的圖像讀入為 QImage 類型,而后程序里將其轉(zhuǎn)為 QPixmap 類型
- 正常方法設(shè)置即可,超清圖像完美被加載
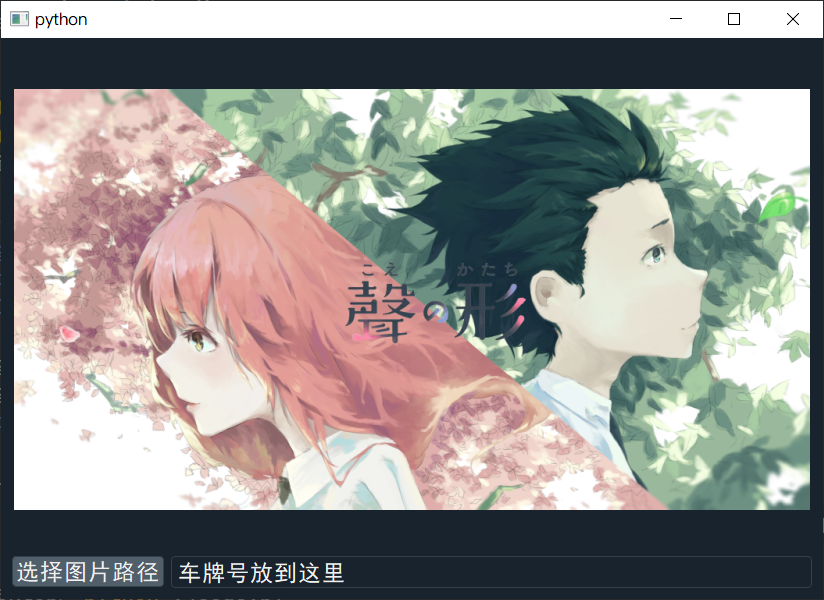
以上就是PyQt5 顯示超清高分辨率圖片的方法的詳細(xì)內(nèi)容,更多關(guān)于PyQt5 顯示超清高分辨率圖片的資料請(qǐng)關(guān)注腳本之家其它相關(guān)文章!
您可能感興趣的文章:- PyQt5 實(shí)現(xiàn)給無(wú)邊框widget窗口添加背景圖片
- opencv+pyQt5實(shí)現(xiàn)圖片閾值編輯器/尋色塊閾值利器
- 使用PyQt5實(shí)現(xiàn)圖片查看器的示例代碼
- python GUI框架pyqt5 對(duì)圖片進(jìn)行流式布局的方法(瀑布流flowlayout)
- python GUI庫(kù)圖形界面開發(fā)之PyQt5圖片顯示控件QPixmap詳細(xì)使用方法與實(shí)例
- pyqt5 使用cv2 顯示圖片,攝像頭的實(shí)例
- pyqt5讓圖片自適應(yīng)QLabel大小上以及移除已顯示的圖片方法
- pyqt5實(shí)現(xiàn)繪制ui,列表窗口,滾動(dòng)窗口顯示圖片的方法
- PyQt5 QTable插入圖片并動(dòng)態(tài)更新的實(shí)例
- PyQt5 對(duì)圖片進(jìn)行縮放的實(shí)例