目錄
- 假想場景
- 基本思路
- pywinauto方案
- win32gui方案
- 更一般的方案
利用Python進行Excel自動化操作的過程中,尤其是涉及VBA時,可能遇到消息框/彈窗(MsgBox)。此時需要人為響應,否則代碼卡死直至超時 [^1] [^2]。根本的解決方法是VBA代碼中不要出現類似彈窗,但有時我們無權修改被操作的Excel文件,例如這是我們進行自動化測試的對象。所以本文記錄從代碼角度解決此類問題的方法。
假想場景
使用xlwings(或者其他自動化庫)打開Excel文件test.xlsm,讀取Sheet1!A1單元格內容。很簡單的一個操作:
import xlwings as xw
wb = xw.Book('test.xlsm')
msg = wb.sheets('Sheet1').range('A1').value
print(msg)
wb.close()
然而不幸的是,打開工作簿時進行了熱情的歡迎儀式:
Private Sub Workbook_Open()
MsgBox "Welcome"
MsgBox "to open"
MsgBox "this file."
End Sub
第一個彈窗Welcome就卡住了Excel,Python代碼相應卡死在第一行。
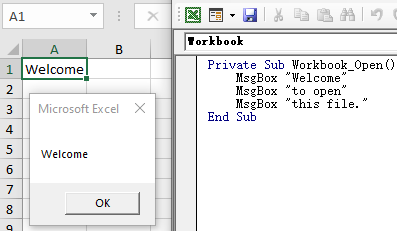
基本思路
主程序中不可能直接處理或者繞過此類問題,也不能奢望有人隨時蹲守點擊下一步——那就開啟一個子線程來護航吧。因此,解決方案是利用子線程監(jiān)聽并隨時關閉彈窗,直到主程序圓滿結束。
解決這個問題,需要以下兩個知識點(基礎知識請課外學習):
- Python多線程(本文采用threading.Thread)
- Python界面自動化庫(本文涉及pywinauto和pywin32)
pywinauto方案
pywinauto顧名思義是Windows界面自動化庫,模擬鼠標和鍵盤操作窗體和控件 [^3]。不同于先獲取句柄再獲取屬性的傳統方式,pywinauto的API更加友好和pythonic。例如,兩行代碼搞定窗口捕捉和點擊:
from pywinauto.application import Application
win = Application(backend="win32").connect(title='Microsoft Excel')
win.Dialog.Button.click()
本文采用自定義線程類的方式,啟動線程后自動執(zhí)行run()函數來完成上述操作。具體代碼如下,注意構造函數中的兩個參數:
- title 需要捕捉的彈窗的標題,例如Excel默認彈窗的標題為Microsoft Excel
- interval 監(jiān)聽的頻率,即每隔多少秒檢查一次
# listener.py
import time
from threading import Thread, Event
from pywinauto.application import Application
class MsgBoxListener(Thread):
def __init__(self, title:str, interval:int):
Thread.__init__(self)
self._title = title
self._interval = interval
self._stop_event = Event()
def stop(self): self._stop_event.set()
@property
def is_running(self): return not self._stop_event.is_set()
def run(self):
while self.is_running:
try:
time.sleep(self._interval)
self._close_msgbox()
except Exception as e:
print(e, flush=True)
def _close_msgbox(self):
'''Close the default Excel MsgBox with title "Microsoft Excel".'''
win = Application(backend="win32").connect(title=self._title)
win.Dialog.Button.click()
if __name__=='__main__':
t = MsgBoxListener('Microsoft Excel', 3)
t.start()
time.sleep(10)
t.stop()
于是,整個過程分為三步:
- 啟動子線程監(jiān)聽彈窗
- 主線程中打開Excel開始自動化操作
- 關閉子線程
import xlwings as xw
from listener import MsgBoxListener
# start listen thread
listener = MsgBoxListener('Microsoft Excel', 3)
listener.start()
# main process as before
wb = xw.Book('test.xlsm')
msg = wb.sheets('Sheet1').range('A1').value
print(msg)
wb.close()
# stop listener thread
listener.stop()
到此問題基本解決,本地運行效果完全達到預期。但我的真實需求是以系統服務方式在服務器上進行Excel文件自動化測試,后續(xù)發(fā)現,當以系統服務方式運行時,pywinauto竟然捕捉不到彈窗!這或許是pywinauto一個潛在的問題 [^4]。
win32gui方案
那就只好轉向相對底層的win32gui,所幸完美解決了上述問題。
win32gui是pywin32庫的一部分,所以實際安裝命令是:
整個方案和前文描述完全一致,只是替換MsgBoxListener類中關閉彈窗的方法:
import win32gui, win32con
def _close_msgbox(self):
# find the top window by title
hwnd = win32gui.FindWindow(None, self._title)
if not hwnd: return
# find child button
h_btn = win32gui.FindWindowEx(hwnd, None,'Button', None)
if not h_btn: return
# show text
text = win32gui.GetWindowText(h_btn)
print(text)
# click button
win32gui.PostMessage(h_btn, win32con.WM_LBUTTONDOWN, None, None)
time.sleep(0.2)
win32gui.PostMessage(h_btn, win32con.WM_LBUTTONUP, None, None)
time.sleep(0.2)
更一般的方案
更一般地,當同時存在默認標題和自定義標題的彈窗時,就不便于采用標題方式進行捕捉了。例如
MsgBox "Message with default title.", vbInformation,
MsgBox "Message with title My App 1", vbInformation, "My App 1"
MsgBox "Message with title My App 2", vbInformation, "My App 2"
那就擴大搜索范圍,依次點擊所有包含確定性描述的按鈕(例如OK,Yes,Confirm)來關閉彈窗。同理替換MsgBoxListener類的_close_msgbox()方法(同時構造函數中不再需要title參數):
def _close_msgbox(self):
'''Click any button ("OK", "Yes" or "Confirm") to close message box.'''
# get handles of all top windows
h_windows = []
win32gui.EnumWindows(lambda hWnd, param: param.append(hWnd), h_windows)
# check each window
for h_window in h_windows:
# get child button with text OK, Yes or Confirm of given window
h_btn = win32gui.FindWindowEx(h_window, None,'Button', None)
if not h_btn: continue
# check button text
text = win32gui.GetWindowText(h_btn)
if not text.lower() in ('ok', 'yes', 'confirm'): continue
# click button
win32gui.PostMessage(h_btn, win32con.WM_LBUTTONDOWN, None, None)
time.sleep(0.2)
win32gui.PostMessage(h_btn, win32con.WM_LBUTTONUP, None, None)
time.sleep(0.2)
最后,實例演示結束全文,以后再也不用擔心意外彈窗了。
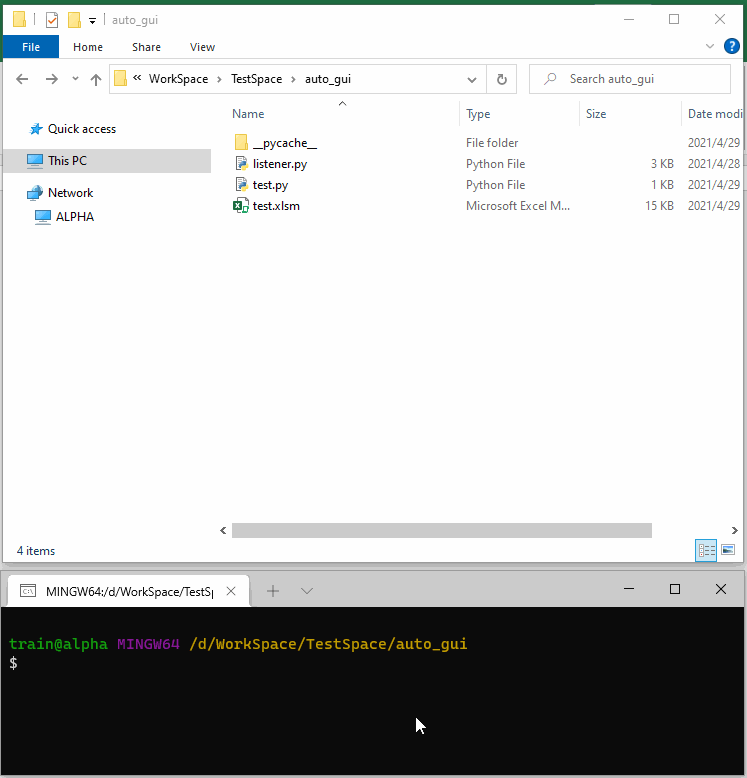
以上就是如何用 Python 子進程關閉 Excel 自動化中的彈窗的詳細內容,更多關于Python 子進程關閉 Excel 彈窗的資料請關注腳本之家其它相關文章!
您可能感興趣的文章:- python子線程如何有序執(zhí)行
- 解決python父線程關閉后子線程不關閉問題
- Python 多線程,threading模塊,創(chuàng)建子線程的兩種方式示例
- python清理子進程機制剖析
- python使用Queue在多個子進程間交換數據的方法
- Python的子線程和子進程是如何手動結束的?