目錄
- 一、初始化主界面
- 二、第一種進度條
- 三、第二種進度條
- 四、第三種進度條
- 五、第四種進度條
- 六、綜合案例
一、初始化主界面
import pygame
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的進度條顯示V1.0")
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
clock.tick(30)
pygame.display.flip()
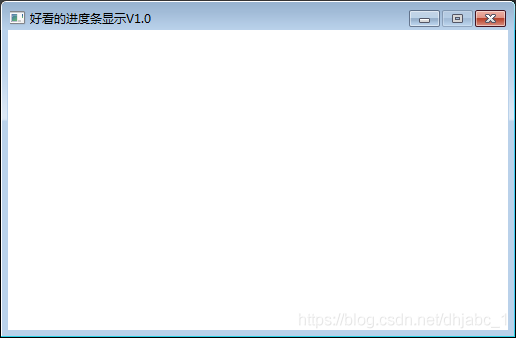
二、第一種進度條
(一)核心代碼
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step,20))
(二)設(shè)置步長,并循環(huán)遞增
(三)完整代碼
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的進度條顯示V1.0")
clock = pygame.time.Clock()
step = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % 490,20))
step += 1
clock.tick(60)
pygame.display.flip()
(四)運行效果

三、第二種進度條
(一)核心代碼
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % 490,20))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % 490)/490*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
(二)完整代碼
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的進度條顯示V1.0")
clock = pygame.time.Clock()
step = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % 490,20))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % 490)/490*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
step += 1
clock.tick(60)
pygame.display.flip()
(三)運行結(jié)果
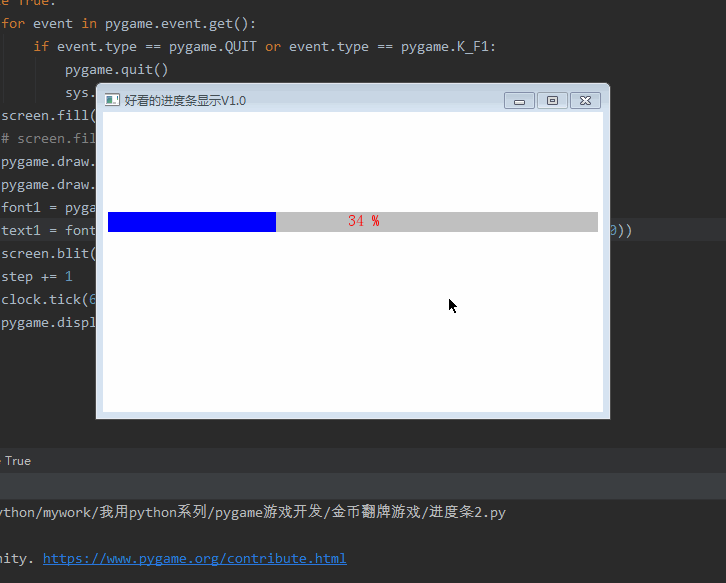
四、第三種進度條
(一)核心代碼
pygame.draw.rect(screen,(192,192,192),(5,100,length+10,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % length,20))
pygame.draw.circle(screen,(0,0,255),(step % length,110),10)
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
(二)完整代碼
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的進度條顯示V1.0")
clock = pygame.time.Clock()
step = 0
length = 480
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
pygame.draw.rect(screen,(192,192,192),(5,100,length+10,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % length,20))
pygame.draw.circle(screen,(0,0,255),(step % length,110),10)
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
step += 1
clock.tick(60)
pygame.display.flip()
(三)運行效果
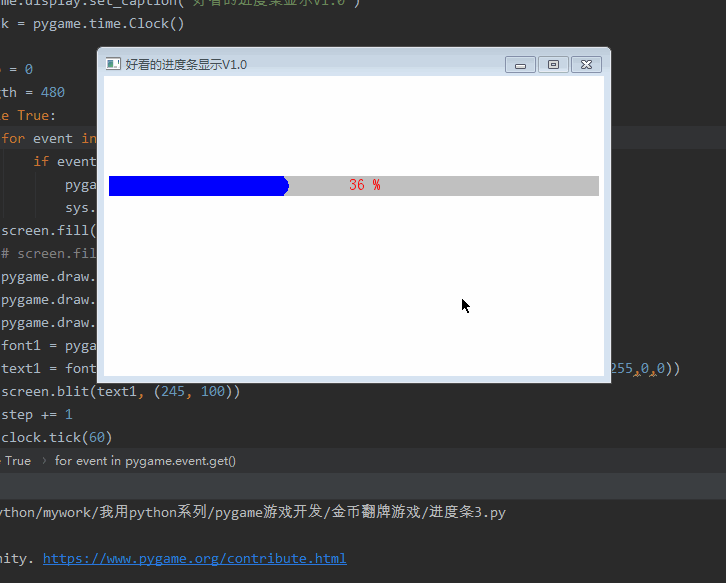
五、第四種進度條
(一)加載圖片資源
picture = pygame.transform.scale(pygame.image.load('score/5.png'), (20, 20))
(二)畫進度條
pygame.draw.rect(screen,(192,192,192),(5,100,length+10,20))
pygame.draw.rect(screen,(251,174,63),(5,100,step % length,20))
(三)畫圖片資源
screen.blit(picture,(step%length,100))
(四)畫文字
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
(五)完整代碼
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的進度條顯示V1.0")
clock = pygame.time.Clock()
picture = pygame.transform.scale(pygame.image.load('score/5.png'), (20, 20))
step = 0
length = 480
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
pygame.draw.rect(screen,(192,192,192),(5,100,length+10,20))
pygame.draw.rect(screen,(251,174,63),(5,100,step % length,20))
screen.blit(picture,(step%length,100))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 100))
step += 1
clock.tick(60)
pygame.display.flip()
(六)運行效果

六、綜合案例
(一)完整代碼
import pygame,sys
pygame.init()
screen = pygame.display.set_mode((500,300))
pygame.display.set_caption("好看的進度條顯示V1.0")
clock = pygame.time.Clock()
picture = pygame.transform.scale(pygame.image.load('score/5.png'), (20, 20))
step = 0
length = 480
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT or event.type == pygame.K_F1:
pygame.quit()
sys.exit()
screen.fill((255,255,255))
# screen.fill((0,0,0))
# 第一種
pygame.draw.rect(screen,(192,192,192),(5,100,490,20))
pygame.draw.rect(screen,(0,0,255),(5,100,step % 490,20))
# 第二種
pygame.draw.rect(screen,(192,192,192),(5,150,490,20))
pygame.draw.rect(screen,(0,0,255),(5,150,step % 490,20))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % 490)/490*100)), True, (255,0,0))
screen.blit(text1, (245, 150))
# 第三種
pygame.draw.rect(screen,(192,192,192),(5,200,length+10,20))
pygame.draw.rect(screen,(0,0,255),(5,200,step % length,20))
pygame.draw.circle(screen,(0,0,255),(step % length,210),10)
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 200))
# 第四種
pygame.draw.rect(screen,(192,192,192),(5,250,length+10,20))
pygame.draw.rect(screen,(251,174,63),(5,250,step % length,20))
screen.blit(picture,(step%length,250))
font1 = pygame.font.Font(r'C:\Windows\Fonts\simsun.ttc', 16)
text1 = font1.render('%s %%' % str(int((step % length)/length*100)), True, (255,0,0))
screen.blit(text1, (245, 250))
step += 1
clock.tick(60)
pygame.display.flip()
(二)運行效果
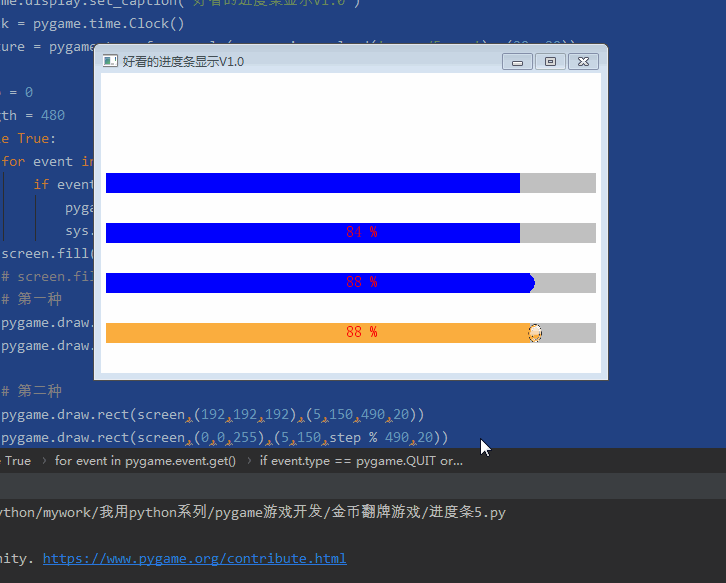
OK,寫完,本博文純屬科普貼,技術(shù)含量不高,入門級別,大家喜歡就好。
而且里面代碼相對比較簡單,也沒有考慮優(yōu)化,大家在實操過程中可以優(yōu)化完善,并反饋給我一起進步。
到此這篇關(guān)于Python趣味挑戰(zhàn)之教你用pygame畫進度條的文章就介紹到這了,更多相關(guān)pygame畫進度條內(nèi)容請搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- Python趣味挑戰(zhàn)之pygame實現(xiàn)無敵好看的百葉窗動態(tài)效果
- Python趣味挑戰(zhàn)之用pygame實現(xiàn)簡單的金幣旋轉(zhuǎn)效果
- Python3+Pygame實現(xiàn)射擊游戲完整代碼
- python 基于pygame實現(xiàn)俄羅斯方塊
- python pygame 憤怒的小鳥游戲示例代碼
- Python3.9.0 a1安裝pygame出錯解決全過程(小結(jié))
- python之pygame模塊實現(xiàn)飛機大戰(zhàn)完整代碼
- Python使用Pygame繪制時鐘
- Python3.8安裝Pygame教程步驟詳解
- python pygame入門教程