先看偉大的高斯分布(Gaussian Distribution)的概率密度函數(shù)(probability density function):
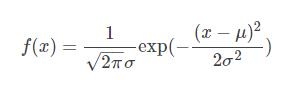
對應(yīng)于numpy中:
numpy.random.normal(loc=0.0, scale=1.0, size=None)
參數(shù)的意義為:
loc
:float
此概率分布的均值(對應(yīng)著整個分布的中心centre)
scale
:float
此概率分布的標(biāo)準(zhǔn)差(對應(yīng)于分布的寬度,scale越大越矮胖,scale越小,越瘦高)
size
:int or tuple of ints
輸出的shape,默認(rèn)為None,只輸出一個值
我們更經(jīng)常會用到的np.random.randn(size)所謂標(biāo)準(zhǔn)正態(tài)分布
對應(yīng)于np.random.normal(loc=0, scale=1, size)。
采樣(sampling)
# 從某一分布(由均值和標(biāo)準(zhǔn)差標(biāo)識)中獲得樣本
mu, sigma = 0, .1
s = np.random.normal(loc=mu, scale=sigma, size=1000)
也可使用scipy庫中的相關(guān)api(這里的類與函數(shù)更符合數(shù)理統(tǒng)計中的直覺):
import scipy.stats as st
mu, sigma = 0, .1
s = st.norm(mu, sigma).rvs(1000)
校驗均值和方差:
>>> abs(mu np.mean(s)) .01
True
>>> abs(sigma-np.std(s, ddof=1)) .01
True
# ddof,delta degrees of freedom,表示自由度
# 一般取1,表示無偏估計,
擬合
我們看使用matplotlib.pyplot便捷而強大的語法如何進行高斯分布的擬合:
import matplotlib.pyplot as plt
count, bins, _ = plt.hist(s, 30, normed=True)
# normed是進行擬合的關(guān)鍵
# count統(tǒng)計某一bin出現(xiàn)的次數(shù),在Normed為True時,可能其值會略有不同
plt.plot(bins, 1./(np.sqrt(2*np.pi)*sigma)*np.exp(-(bins-mu)**2/(2*sigma**2), lw=2, c='r')
plt.show()
或者:
s_fit = np.linspace(s.min(), s.max())
plt.plot(s_fit, st.norm(mu, sigma).pdf(s_fit), lw=2, c='r')
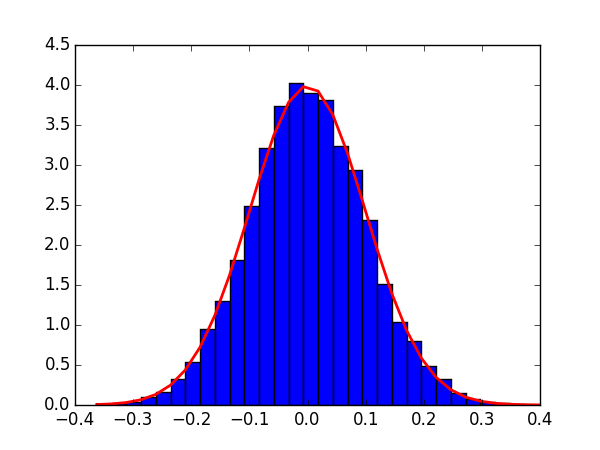
np.random.normal()的含義及實例
這是個隨機產(chǎn)生正態(tài)分布的函數(shù)。(normal 表正態(tài))
先看一下官方解釋:
有三個參數(shù)
loc
:正態(tài)分布的均值,對應(yīng)著這個分布的中心.代表下圖的μ
scale
:正態(tài)分布的標(biāo)準(zhǔn)差,對應(yīng)分布的寬度,scale越大,正態(tài)分布的曲線 越矮胖,scale越小,曲線越高瘦。 代表下圖的σ
size
:你輸入數(shù)據(jù)的shape,例子:
下面展示一些 內(nèi)聯(lián)代碼片。
// An highlighted block
a=np.random.normal(0, 1, (2, 4))
print(a)
輸出:
[[-0.29217334 0.41371571 1.26816017 0.46474676]
[ 1.33271487 0.80162296 0.47974157 -1.49748788]]
看這個圖直觀些:

以下為官方文檔:

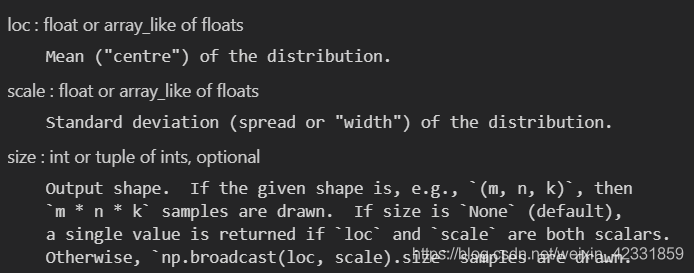
以上為個人經(jīng)驗,希望能給大家一個參考,也希望大家多多支持腳本之家。
您可能感興趣的文章:- python numpy之np.random的隨機數(shù)函數(shù)使用介紹
- Numpy中np.random.rand()和np.random.randn() 用法和區(qū)別詳解
- Numpy之random函數(shù)使用學(xué)習(xí)