目錄
- 1、連接PG庫(kù)
- 2、連接MySQL
- 2.1 連接數(shù)據(jù)庫(kù)
- 2.2 創(chuàng)建數(shù)據(jù)庫(kù)和表
- 2.3 插入數(shù)據(jù)
- 2.4 數(shù)據(jù)庫(kù)查詢操作
- 2.5 數(shù)據(jù)庫(kù)更新操作
- 2.6 刪除數(shù)據(jù)操作
- 3、連接Mongo庫(kù)
- 3.1 判讀庫(kù)是否存在
- 3.2 創(chuàng)建集合(表)
- 3.3 插入集合
- 3.4 返回 _id 字段
- 3.5 插入多個(gè)文檔
- 3.6 插入指定 _id 的多個(gè)文檔
- 3.7 查詢一條數(shù)據(jù)
- 3.8 查詢集合中所有數(shù)據(jù)
- 3.9 查詢指定字段的數(shù)據(jù)
- 3.10 根據(jù)指定條件查詢
- 3.11 高級(jí)查詢
- 3.12 使用正則表達(dá)式查詢
- 3.13 返回指定條數(shù)記錄
- 3.14 修改數(shù)據(jù)
- 3.15 排序
- 3.16 刪除數(shù)據(jù)
- 3.17 刪除集合中的所有文檔
- 3.17 刪除集合
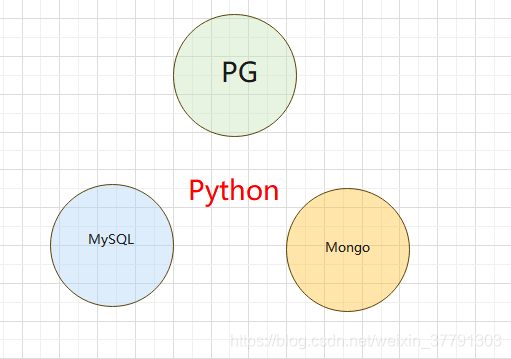
Postgres/Mysql/Mongo是本人在工作中最常用到的數(shù)據(jù)庫(kù)?,F(xiàn)羅列了python操作這三種數(shù)據(jù)庫(kù)的基本操作,只要掌握了基本的操作,再多加訓(xùn)練,其實(shí)可以應(yīng)用的得心應(yīng)手。
1、連接PG庫(kù)
## 導(dǎo)入psycopg2包
import psycopg2
## 連接到一個(gè)給定的數(shù)據(jù)庫(kù)
conn = psycopg2.connect(database="zabbix", user="zabbix",password="zabbix", host="127.0.0.1", port="5432")
## 建立游標(biāo),用來(lái)執(zhí)行數(shù)據(jù)庫(kù)操作
cursor = conn.cursor()
## 執(zhí)行SQL命令
#cursor.execute("CREATE TABLE test_conn(id int, name text)")
#cursor.execute("INSERT INTO test_conn values(1,'haha')")
## 提交SQL命令
#conn.commit()
## 執(zhí)行SQL SELECT命令
cursor.execute("select * from drules;")
## 獲取SELECT返回的元組
rows = cursor.fetchall()
print('druleid|proxy_hostid|name|iprange| delay|nextcheck|status')
for row in rows:
#print(row)
print(row[0],row[1],row[2],row[3],row[4],row[5])
## 關(guān)閉游標(biāo)
cursor.close()
## 關(guān)閉數(shù)據(jù)庫(kù)連接
conn.close()
2、連接MySQL
2.1 連接數(shù)據(jù)庫(kù)
連接數(shù)據(jù)庫(kù)前,請(qǐng)先確認(rèn)以下事項(xiàng):
- 您已經(jīng)創(chuàng)建了數(shù)據(jù)庫(kù) TESTDB.
- 在TESTDB數(shù)據(jù)庫(kù)中您已經(jīng)創(chuàng)建了表 EMPLOYEE
- EMPLOYEE表字段為FIRST_NAME, LAST_NAME, AGE, SEX 和 INCOME。
- 連接數(shù)據(jù)庫(kù)TESTDB使用的用戶名為"testuser" ,密碼為 “test123”,你可以可以自己設(shè)定或者直接使用root用戶名及其密碼,Mysql數(shù)據(jù)庫(kù)用戶授權(quán)請(qǐng)使用Grant命令。
- 在你的機(jī)子上已經(jīng)安裝了 Python MySQLdb 模塊。 如果您對(duì)sql語(yǔ)句不熟悉,可以訪問(wèn)我們的 SQL基礎(chǔ)教程
#!/usr/bin/python
# -*- coding: UTF-8 -*-
import MySQLdb
# 打開(kāi)數(shù)據(jù)庫(kù)連接
db = MySQLdb.connect("localhost", "testuser", "test123", "TESTDB", charset='utf8' )
# 使用cursor()方法獲取操作游標(biāo)
cursor = db.cursor()
# 使用execute方法執(zhí)行SQL語(yǔ)句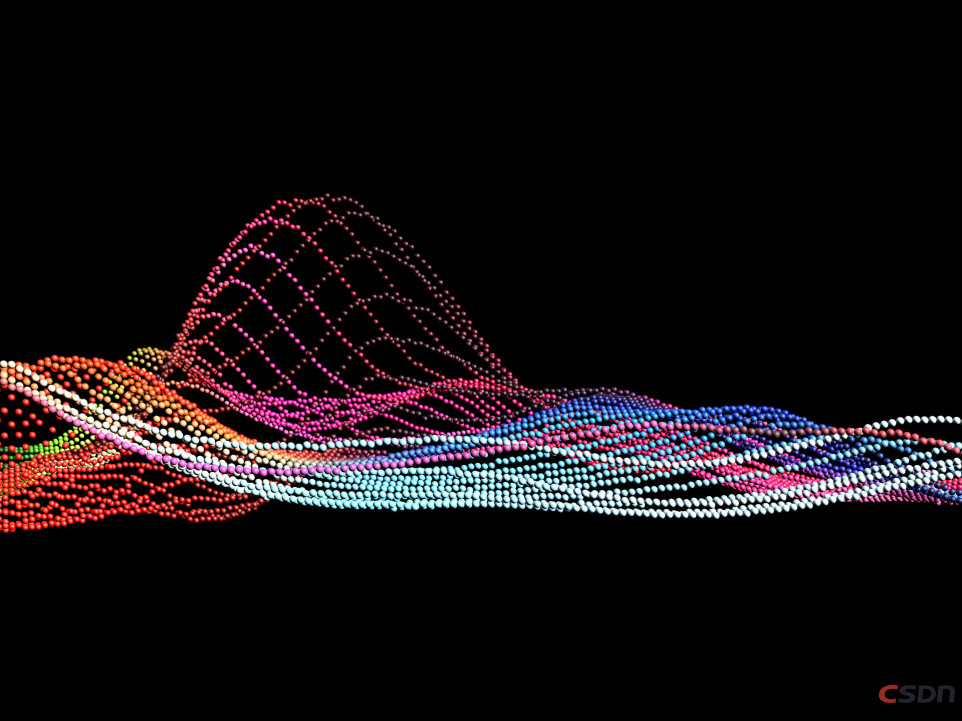
cursor.execute("SELECT VERSION()")
# 使用 fetchone() 方法獲取一條數(shù)據(jù)
data = cursor.fetchone()
print "Database version : %s " % data
# 關(guān)閉數(shù)據(jù)庫(kù)連接
db.close()
2.2 創(chuàng)建數(shù)據(jù)庫(kù)和表
#!/usr/bin/python
# -*- coding: UTF-8 -*-
import MySQLdb
# 打開(kāi)數(shù)據(jù)庫(kù)連接
db = MySQLdb.connect("localhost", "testuser", "test123", "TESTDB", charset='utf8' )
# 使用cursor()方法獲取操作游標(biāo)
cursor = db.cursor()
# 如果數(shù)據(jù)表已經(jīng)存在使用 execute() 方法刪除表。
cursor.execute("DROP TABLE IF EXISTS EMPLOYEE")
# 創(chuàng)建數(shù)據(jù)表SQL語(yǔ)句
sql = """CREATE TABLE EMPLOYEE (
FIRST_NAME CHAR(20) NOT NULL,
LAST_NAME CHAR(20),
AGE INT,
SEX CHAR(1),
INCOME FLOAT )"""
cursor.execute(sql)
# 關(guān)閉數(shù)據(jù)庫(kù)連接
db.close()
2.3 插入數(shù)據(jù)
#!/usr/bin/python
# -*- coding: UTF-8 -*-
import MySQLdb
# 打開(kāi)數(shù)據(jù)庫(kù)連接
db = MySQLdb.connect("localhost", "testuser", "test123", "TESTDB", charset='utf8' )
# 使用cursor()方法獲取操作游標(biāo)
cursor = db.cursor()
# SQL 插入語(yǔ)句
sql = """INSERT INTO EMPLOYEE(FIRST_NAME,
LAST_NAME, AGE, SEX, INCOME)
VALUES ('Mac', 'Mohan', 20, 'M', 2000)"""
try:
# 執(zhí)行sql語(yǔ)句
cursor.execute(sql)
# 提交到數(shù)據(jù)庫(kù)執(zhí)行
db.commit()
except:
# Rollback in case there is any error
db.rollback()
# 關(guān)閉數(shù)據(jù)庫(kù)連接
db.close()
#!/usr/bin/python
# -*- coding: UTF-8 -*-
import MySQLdb
# 打開(kāi)數(shù)據(jù)庫(kù)連接
db = MySQLdb.connect("localhost", "testuser", "test123", "TESTDB", charset='utf8' )
# 使用cursor()方法獲取操作游標(biāo)
cursor = db.cursor()
# SQL 插入語(yǔ)句
sql = "INSERT INTO EMPLOYEE(FIRST_NAME, \
LAST_NAME, AGE, SEX, INCOME) \
VALUES (%s, %s, %s, %s, %s )" % \
('Mac', 'Mohan', 20, 'M', 2000)
try:
# 執(zhí)行sql語(yǔ)句
cursor.execute(sql)
# 提交到數(shù)據(jù)庫(kù)執(zhí)行
db.commit()
except:
# 發(fā)生錯(cuò)誤時(shí)回滾
db.rollback()
# 關(guān)閉數(shù)據(jù)庫(kù)連接
db.close()
2.4 數(shù)據(jù)庫(kù)查詢操作
Python查詢Mysql使用 fetchone() 方法獲取單條數(shù)據(jù), 使用fetchall() 方法獲取多條數(shù)據(jù)。
- fetchone(): 該方法獲取下一個(gè)查詢結(jié)果集。結(jié)果集是一個(gè)對(duì)象
- fetchall():接收全部的返回結(jié)果行.
- rowcount: 這是一個(gè)只讀屬性,并返回執(zhí)行execute()方法后影響的行數(shù)。
#!/usr/bin/python
# -*- coding: UTF-8 -*-
import MySQLdb
# 打開(kāi)數(shù)據(jù)庫(kù)連接
db = MySQLdb.connect("localhost", "testuser", "test123", "TESTDB", charset='utf8' )
# 使用cursor()方法獲取操作游標(biāo)
cursor = db.cursor()
# SQL 查詢語(yǔ)句
sql = "SELECT * FROM EMPLOYEE \
WHERE INCOME > %s" % (1000)
try:
# 執(zhí)行SQL語(yǔ)句
cursor.execute(sql)
# 獲取所有記錄列表
results = cursor.fetchall()
for row in results:
fname = row[0]
lname = row[1]
age = row[2]
sex = row[3]
income = row[4]
# 打印結(jié)果
print "fname=%s,lname=%s,age=%s,sex=%s,income=%s" % \
(fname, lname, age, sex, income )
except:
print "Error: unable to fecth data"
# 關(guān)閉數(shù)據(jù)庫(kù)連接
db.close()
2.5 數(shù)據(jù)庫(kù)更新操作
#!/usr/bin/python
# -*- coding: UTF-8 -*-
import MySQLdb
# 打開(kāi)數(shù)據(jù)庫(kù)連接
db = MySQLdb.connect("localhost", "testuser", "test123", "TESTDB", charset='utf8' )
# 使用cursor()方法獲取操作游標(biāo)
cursor = db.cursor()
# SQL 更新語(yǔ)句
sql = "UPDATE EMPLOYEE SET AGE = AGE + 1 WHERE SEX = '%c'" % ('M')
try:
# 執(zhí)行SQL語(yǔ)句
cursor.execute(sql)
# 提交到數(shù)據(jù)庫(kù)執(zhí)行
db.commit()
except:
# 發(fā)生錯(cuò)誤時(shí)回滾
db.rollback()
# 關(guān)閉數(shù)據(jù)庫(kù)連接
db.close()
2.6 刪除數(shù)據(jù)操作
#!/usr/bin/python
# -*- coding: UTF-8 -*-
import MySQLdb
# 打開(kāi)數(shù)據(jù)庫(kù)連接
db = MySQLdb.connect("localhost", "testuser", "test123", "TESTDB", charset='utf8' )
# 使用cursor()方法獲取操作游標(biāo)
cursor = db.cursor()
# SQL 刪除語(yǔ)句
sql = "DELETE FROM EMPLOYEE WHERE AGE > %s" % (20)
try:
# 執(zhí)行SQL語(yǔ)句
cursor.execute(sql)
# 提交修改
db.commit()
except:
# 發(fā)生錯(cuò)誤時(shí)回滾
db.rollback()
# 關(guān)閉連接
db.close()
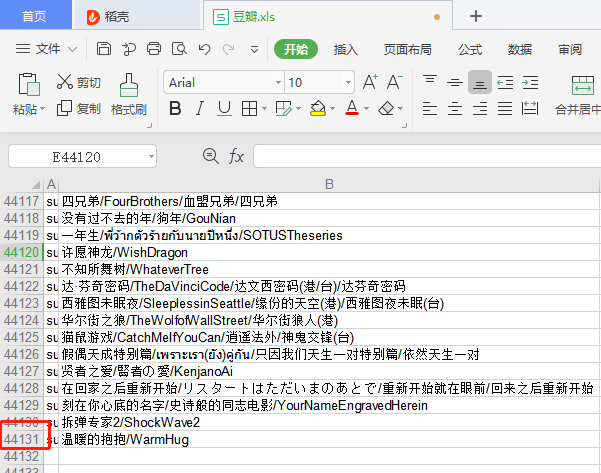
3、連接Mongo庫(kù)
3.1 判讀庫(kù)是否存在
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
dblist = myclient.list_database_names()
# dblist = myclient.database_names()
if "runoobdb" in dblist:
print("數(shù)據(jù)庫(kù)已存在!")
3.2 創(chuàng)建集合(表)
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
collist = mydb. list_collection_names()
# collist = mydb.collection_names()
if "sites" in collist: # 判斷 sites 集合是否存在
print("集合已存在!")
else:
mycol = mydb["sites"]
3.3 插入集合
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
mydict = { "name": "RUNOOB", "alexa": "10000", "url": "https://www.runoob.com" }
x = mycol.insert_one(mydict)
print(x)
print(x)
3.4 返回 _id 字段
insert_one() 方法返回 InsertOneResult 對(duì)象,該對(duì)象包含 inserted_id 屬性,它是插入文檔的 id 值。
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient('mongodb://localhost:27017/')
mydb = myclient['runoobdb']
mycol = mydb["sites"]
mydict = { "name": "Google", "alexa": "1", "url": "https://www.google.com" }
x = mycol.insert_one(mydict)
print(x.inserted_id)
3.5 插入多個(gè)文檔
集合中插入多個(gè)文檔使用 insert_many() 方法,該方法的第一參數(shù)是字典列表。
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
mylist = [
{ "name": "Taobao", "alexa": "100", "url": "https://www.taobao.com" },
{ "name": "QQ", "alexa": "101", "url": "https://www.qq.com" },
{ "name": "Facebook", "alexa": "10", "url": "https://www.facebook.com" },
{ "name": "知乎", "alexa": "103", "url": "https://www.zhihu.com" },
{ "name": "Github", "alexa": "109", "url": "https://www.github.com" }
]
x = mycol.insert_many(mylist)
# 輸出插入的所有文檔對(duì)應(yīng)的 _id 值
print(x.inserted_ids)
3.6 插入指定 _id 的多個(gè)文檔
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["site2"]
mylist = [
{ "_id": 1, "name": "RUNOOB", "cn_name": "菜鳥(niǎo)教程"},
{ "_id": 2, "name": "Google", "address": "Google 搜索"},
{ "_id": 3, "name": "Facebook", "address": "臉書"},
{ "_id": 4, "name": "Taobao", "address": "淘寶"},
{ "_id": 5, "name": "Zhihu", "address": "知乎"}
]
x = mycol.insert_many(mylist)
# 輸出插入的所有文檔對(duì)應(yīng)的 _id 值
print(x.inserted_ids)
3.7 查詢一條數(shù)據(jù)
使用 find_one() 方法來(lái)查詢集合中的一條數(shù)據(jù)。
查詢 sites 文檔中的第一條數(shù)據(jù):
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
x = mycol.find_one()
print(x)
3.8 查詢集合中所有數(shù)據(jù)
find() 方法可以查詢集合中的所有數(shù)據(jù),類似 SQL 中的 SELECT * 操作。
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
for x in mycol.find():
print(x)
3.9 查詢指定字段的數(shù)據(jù)
可以使用 find() 方法來(lái)查詢指定字段的數(shù)據(jù),將要返回的字段對(duì)應(yīng)值設(shè)置為 1。
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
for x in mycol.find({},{ "_id": 0, "name": 1, "alexa": 1 }):
print(x)
除了 _id 你不能在一個(gè)對(duì)象中同時(shí)指定 0 和 1,如果你設(shè)置了一個(gè)字段為 0,則其他都為 1,反之亦然。
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
for x in mycol.find({},{ "alexa": 0 }):
print(x)
3.10 根據(jù)指定條件查詢
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
myquery = { "name": "RUNOOB" }
mydoc = mycol.find(myquery)
for x in mydoc:
print(x)
3.11 高級(jí)查詢
以下實(shí)例用于讀取 name 字段中第一個(gè)字母 ASCII 值大于 “H” 的數(shù)據(jù),大于的修飾符條件為 {"$gt": “H”} :
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
myquery = { "name": { "$gt": "H" } }
mydoc = mycol.find(myquery)
for x in mydoc:
print(x)
3.12 使用正則表達(dá)式查詢
正則表達(dá)式修飾符只用于搜索字符串的字段。
以下實(shí)例用于讀取 name 字段中第一個(gè)字母為 “R” 的數(shù)據(jù),正則表達(dá)式修飾符條件為 {"$regex": “^R”} :
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
myquery = { "name": { "$regex": "^R" } }
mydoc = mycol.find(myquery)
for x in mydoc:
print(x)
3.13 返回指定條數(shù)記錄
如果我們要對(duì)查詢結(jié)果設(shè)置指定條數(shù)的記錄可以使用 limit() 方法,該方法只接受一個(gè)數(shù)字參數(shù)。
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
myresult = mycol.find().limit(3)
# 輸出結(jié)果
for x in myresult:
print(x)
3.14 修改數(shù)據(jù)
以在 MongoDB 中使用 update_one() 方法修改文檔中的記錄。該方法第一個(gè)參數(shù)為查詢的條件,第二個(gè)參數(shù)為要修改的字段。
如果查找到的匹配數(shù)據(jù)多于一條,則只會(huì)修改第一條。
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
myquery = { "alexa": "10000" }
newvalues = { "$set": { "alexa": "12345" } }
mycol.update_one(myquery, newvalues)
# 輸出修改后的 "sites" 集合
for x in mycol.find():
print(x)
update_one() 方法只能修匹配到的第一條記錄,如果要修改所有匹配到的記錄,可以使用 update_many()。
以下實(shí)例將查找所有以 F 開(kāi)頭的 name 字段,并將匹配到所有記錄的 alexa 字段修改為 123:
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
myquery = { "name": { "$regex": "^F" } }
newvalues = { "$set": { "alexa": "123" } }
x = mycol.update_many(myquery, newvalues)
print(x.modified_count, "文檔已修改")
3.15 排序
sort() 方法可以指定升序或降序排序。
sort() 方法第一個(gè)參數(shù)為要排序的字段,第二個(gè)字段指定排序規(guī)則,1 為升序,-1 為降序,默認(rèn)為升序。
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
mydoc = mycol.find().sort("alexa")
for x in mydoc:
print(x)
3.16 刪除數(shù)據(jù)
使用 delete_one() 方法來(lái)刪除一個(gè)文檔,該方法第一個(gè)參數(shù)為查詢對(duì)象,指定要?jiǎng)h除哪些數(shù)據(jù)。
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
myquery = { "name": {"$regex": "^F"} }
x = mycol.delete_many(myquery)
print(x.deleted_count, "個(gè)文檔已刪除")
刪除多個(gè)文檔
delete_many() 方法來(lái)刪除多個(gè)文檔,該方法第一個(gè)參數(shù)為查詢對(duì)象,指定要?jiǎng)h除哪些數(shù)據(jù)。
刪除所有 name 字段中以 F 開(kāi)頭的文檔:
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
x = mycol.delete_many({})
print(x.deleted_count, "個(gè)文檔已刪除")
3.17 刪除集合中的所有文檔
delete_many() 方法如果傳入的是一個(gè)空的查詢對(duì)象,則會(huì)刪除集合中的所有文檔:
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
x = mycol.delete_many({})
print(x.deleted_count, "個(gè)文檔已刪除")
3.17 刪除集合
使用 drop() 方法來(lái)刪除一個(gè)集合。
以下實(shí)例刪除了 customers 集合:
#!/usr/bin/python3
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["runoobdb"]
mycol = mydb["sites"]
mycol.drop()
到此這篇關(guān)于Python連接Postgres/Mysql/Mongo數(shù)據(jù)庫(kù)基本操作的文章就介紹到這了,更多相關(guān)Python連接數(shù)據(jù)庫(kù)內(nèi)容請(qǐng)搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- 將Python腳本打包成MACOSAPP程序過(guò)程
- [項(xiàng)目布局配置]Nosql與PythonWeb-Flask框架組合
- Python使用signal定時(shí)結(jié)束AsyncIOScheduler任務(wù)的問(wèn)題
- python生成可執(zhí)行exe控制Microsip自動(dòng)填寫號(hào)碼并撥打功能
- Python之os模塊案例詳解