目錄
- 創(chuàng)建數(shù)據(jù)
- torch.empty()
- torch.zeros()
- torch.ones()
- torch.tensor()
- torch.rand()
- 數(shù)學(xué)運(yùn)算
- torch.add()
- torch.sub()
- torch.matmul()
- 索引操作
創(chuàng)建數(shù)據(jù)
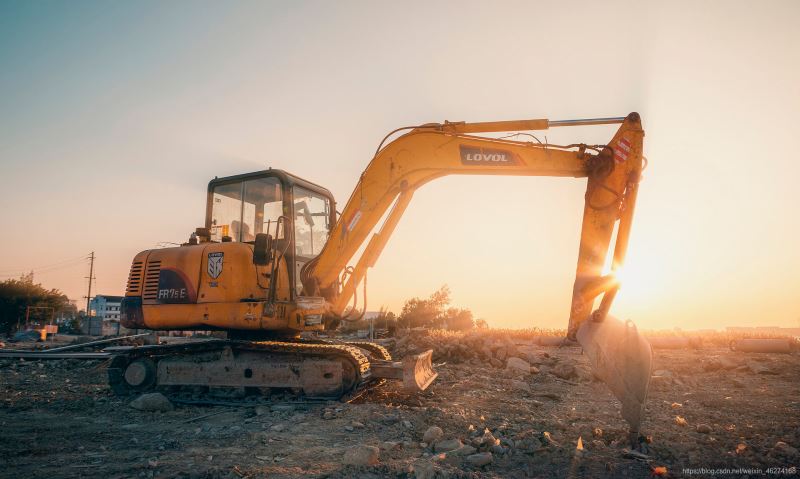
torch.empty()
創(chuàng)建一個(gè)空張量矩陣.
格式:
torch.empty(*size, *, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False, pin_memory=False, memory_format=torch.contiguous_format) → Tensor
參數(shù):
- size: 生成矩陣的形狀, 必選
- dtype: 數(shù)據(jù)類型, 默認(rèn)為 None
例子:
# 創(chuàng)建一個(gè)形狀為[2, 2]的矩陣
a = torch.empty(2, 2)
print(a)
# 創(chuàng)建一個(gè)形狀為[3, 3]的矩陣
b = torch.empty(3, 3)
print(b)
輸出結(jié)果:
tensor([[0., 0.],
[0., 0.]])
tensor([[0., 0., 0.],
[0., 0., 0.],
[0., 0., 0.]])
torch.zeros()
創(chuàng)建一個(gè)全零矩陣.
格式:
torch.zeros(*size, *, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor
參數(shù):
- size: 生成矩陣的形狀, 必選
- dtype: 數(shù)據(jù)類型, 默認(rèn)為 None
例子:
# 創(chuàng)建一個(gè)形狀為[2, 2]的全零數(shù)組
a = torch.zeros([2, 2], dtype=torch.float32)
print(a)
# 創(chuàng)建一個(gè)形狀為[3, 3]的全零數(shù)組
b = torch.zeros([3, 3], dtype=torch.float32)
print(b)
輸出結(jié)果:
tensor([[0., 0.],
[0., 0.]])
tensor([[0., 0., 0.],
[0., 0., 0.],
[0., 0., 0.]])
torch.ones()
創(chuàng)建一個(gè)全一矩陣.
格式:
torch.ones(*size, *, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor
參數(shù):
- size: 生成矩陣的形狀, 必選
- dtype: 數(shù)據(jù)類型, 默認(rèn)為 None
例子:
# 創(chuàng)建一個(gè)形狀為[2, 2]的全一數(shù)組
a = torch.ones([2, 2], dtype=torch.float32)
print(a)
# 創(chuàng)建一個(gè)形狀為[3, 3]的全一數(shù)組
b = torch.ones([3, 3], dtype=torch.float32)
print(b)
輸出結(jié)果:
tensor([[1., 1.],
[1., 1.]])
tensor([[1., 1., 1.],
[1., 1., 1.],
[1., 1., 1.]])
torch.tensor()
通過數(shù)據(jù)創(chuàng)建張量.
格式:
torch.tensor(data, *, dtype=None, device=None, requires_grad=False, pin_memory=False) → Tensor
參數(shù):
- data: 數(shù)據(jù) (數(shù)組, 元組, ndarray, scalar)
- dtype: 數(shù)據(jù)類型, 默認(rèn)為 None
例子:
# 通過數(shù)據(jù)創(chuàng)建張量
array = np.arange(1, 10).reshape(3, 3)
print(array)
print(type(array))
tensor = torch.tensor(array)
print(tensor)
print(type(tensor))
輸出結(jié)果:
[[1 2 3]
[4 5 6]
[7 8 9]]
class 'numpy.ndarray'>
tensor([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]], dtype=torch.int32)
class 'torch.Tensor'>
torch.rand()
創(chuàng)建一個(gè) 0~1 隨機(jī)數(shù)的張量矩陣.
格式:
torch.rand(*size, *, out=None, dtype=None, layout=torch.strided, device=None, requires_grad=False) → Tensor
參數(shù):
- size: 生成矩陣的形狀, 必選
- dtype: 數(shù)據(jù)類型, 默認(rèn)為 None
例子:
# 創(chuàng)建形狀為[2, 2]的隨機(jī)數(shù)矩陣
rand = torch.rand(2, 2)
print(rand)
輸出結(jié)果:
tensor([[0.6209, 0.3424],
[0.3506, 0.7986]])
數(shù)學(xué)運(yùn)算
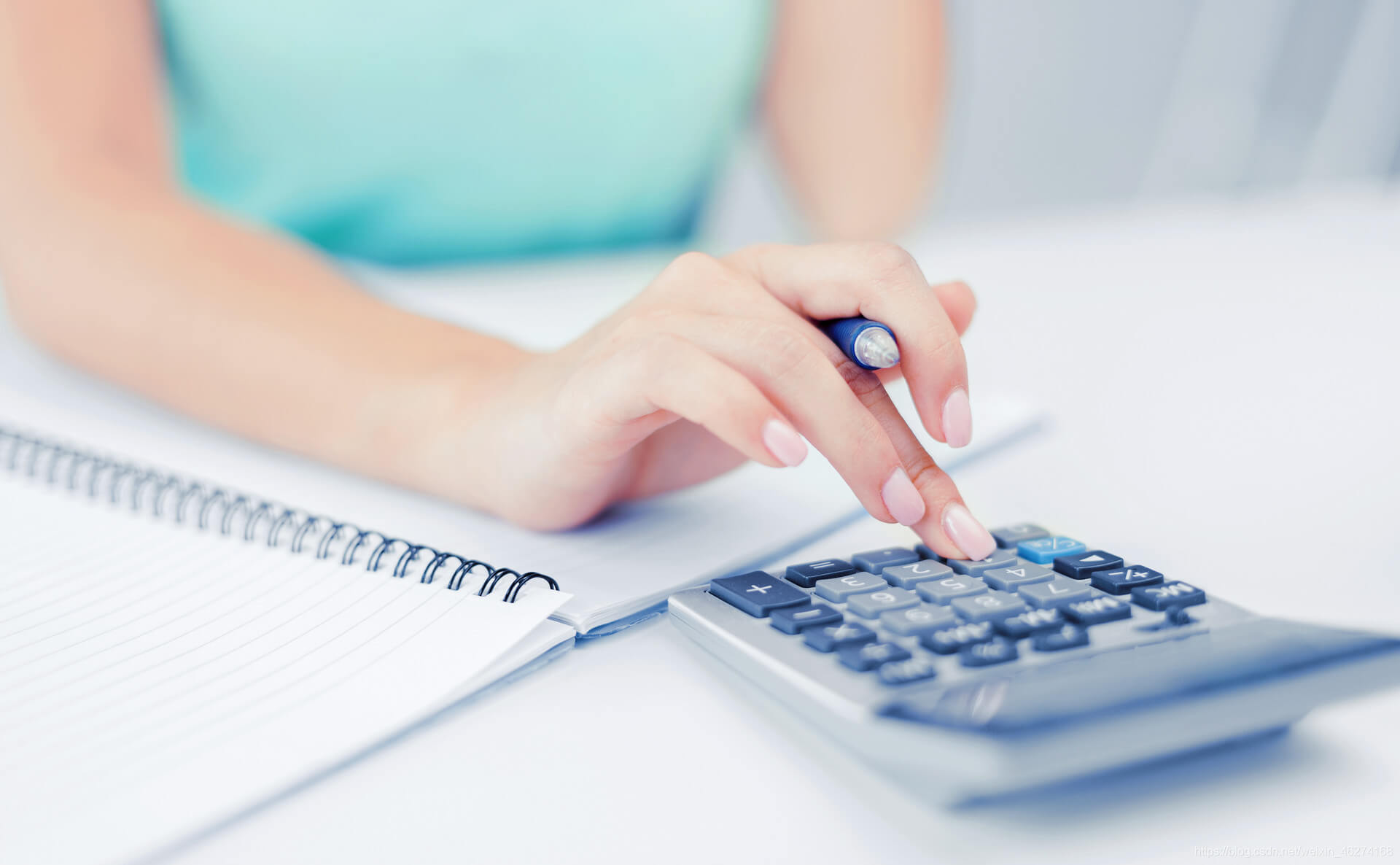
torch.add()
返回相加的張量.
格式:
torch.add(input, other, *, out=None) → Tensor
例子:
# 張量相加
input1 = torch.tensor([[1, 2], [3, 4]])
print(input1)
input2 = torch.tensor([[4, 3], [2, 1]])
print(input2)
output = torch.add(input1, input2)
print(output)
輸出結(jié)果:
tensor([[1, 2],
[3, 4]])
tensor([[4, 3],
[2, 1]])
tensor([[5, 5],
[5, 5]])
注: 相加的張量形狀必須一致, 否則會(huì)報(bào)錯(cuò).
torch.sub()
返回相減的張量.
例子:
# 張量相減
input1 = torch.tensor([[1, 2], [3, 4]])
print(input1)
input2 = torch.tensor([[4, 3], [2, 1]])
print(input2)
output = torch.sub(input1, input2)
print(output)
輸出結(jié)果:
tensor([[1, 2],
[3, 4]])
tensor([[4, 3],
[2, 1]])
tensor([[-3, -1],
[ 1, 3]])
torch.matmul()
例子:
# 張量矩陣相乘
input1 = torch.tensor([[1, 1, 1]])
print(input1)
input2 = torch.tensor([[3], [3], [3]])
print(input2)
output = torch.matmul(input1, input2)
print(output)
輸出結(jié)果:
tensor([[1, 1, 1]])
tensor([[3],
[3],
[3]])
tensor([[9]])
索引操作
索引 (index) 可以幫助我們快速的找到張量中的特定信息.
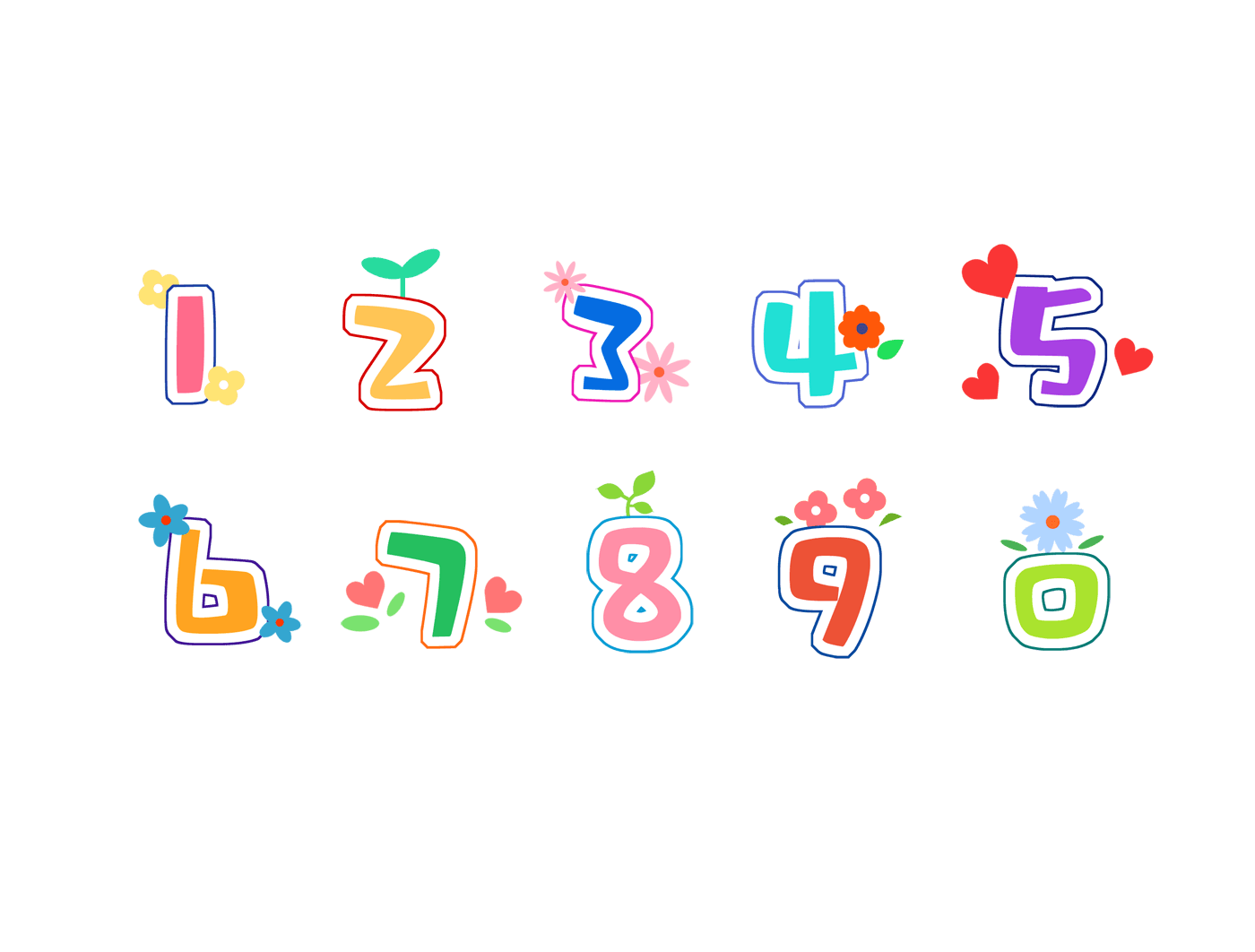
例子:
# 簡(jiǎn)單的索引操作
ones = torch.ones([3, 3])
print(ones[: 2])
print(ones[:, : 2])
調(diào)試輸出:
tensor([[1., 1., 1.],
[1., 1., 1.]])
tensor([[1., 1.],
[1., 1.],
[1., 1.]])
到此這篇關(guān)于PyTorch一小時(shí)掌握之基本操作篇的文章就介紹到這了,更多相關(guān)PyTorch基本操作內(nèi)容請(qǐng)搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- PyTorch一小時(shí)掌握之a(chǎn)utograd機(jī)制篇
- PyTorch一小時(shí)掌握之神經(jīng)網(wǎng)絡(luò)氣溫預(yù)測(cè)篇
- PyTorch一小時(shí)掌握之神經(jīng)網(wǎng)絡(luò)分類篇
- PyTorch一小時(shí)掌握之圖像識(shí)別實(shí)戰(zhàn)篇