本文實例為大家分享了JSP+Servlet實現(xiàn)文件上傳到服務器功能的具體代碼,供大家參考,具體內(nèi)容如下
項目目錄結(jié)構(gòu)大致如下:
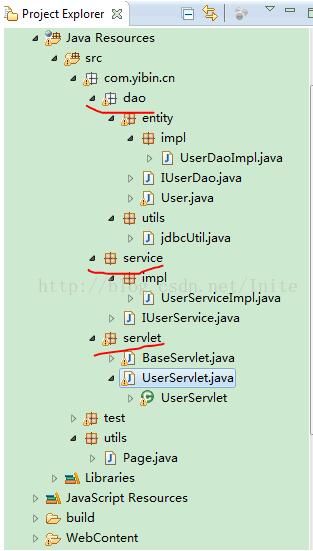
正如我在上圖紅線畫的三個東西:Dao、service、servlet 這三層是主要的結(jié)構(gòu),類似 MVC 架構(gòu),Dao是模型實體類(邏輯層),service是服務層,servlet是視圖層,三者協(xié)作共同完成項目。
這里的User是由user表來定義的一個類,再封裝增刪改查等操作,實現(xiàn)從數(shù)據(jù)庫查詢與插入,修改與刪除等操作,并實現(xiàn)了分頁操作,也實現(xiàn)了將圖片放到服務器上運行的效果。
Dao層:主要實現(xiàn)了User類的定義,接口IUserDao的定義與實現(xiàn)(UserDaoImpl);
service層:直接定義一個接口類IUserService,與IUserDao相似,再實現(xiàn)其接口類UserServiceImpl,直接實例化UserDaoImpl再調(diào)用其方法來實現(xiàn)自己的方法,重用了代碼。詳見代碼吧;
servlet層:起初是將表User 的每個操作方法都定義成一個servlet 去實現(xiàn),雖然簡單,但是太多了,不好管理,于是利用 基類BaseServlet 實現(xiàn)了“反射機制”,通過獲取的 action 參數(shù)自己智能地調(diào)用對應的方法,而UserServlet則具體實現(xiàn)自己的方法,以供調(diào)用,方便許多,詳見之前的博文或下述代碼。
將文件上傳到 tomcat 服務器的編譯后運行的過程的某個文件關(guān)鍵要在每次編譯后手動為其創(chuàng)建該文件夾來存放相應的上傳文件,否則會導致每次重啟 tomcat 服務器后該編譯后的工程覆蓋了原先的,導致上傳文件存放的文件夾不存在,導致代碼找不到該文件夾而報錯,即上傳不成功。如下圖所示:
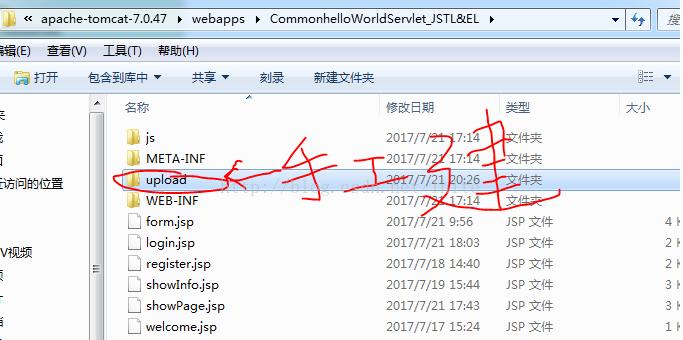
主要是考慮圖片路徑的問題,手工設置路徑肯定不能保證不重復,所以取到上傳圖片的后綴名后利用隨機生成的隨機數(shù)作為圖片名,這樣就不會重復名字了:
String extendedName = picturePath.substring(picturePath.lastIndexOf("."),// 截取從最后一個'.'到字符串結(jié)束的子串。
picturePath.length());
// 把文件名稱重命名為全球唯一的文件名
String uniqueName = UUID.randomUUID().toString();
saveFileName = uniqueName + extendedName;// 拼接路徑名
增加用戶時代碼如下:
// 增
public void add(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
System.out.println("add方法被調(diào)用");
// 獲取數(shù)據(jù)
int id = 0;
String username = null;
String password = null;
String sex = null;
Date birthday = null;
String address = null;
String saveFileName = null;
String picturePath = null;
// 得到表單是否以enctype="multipart/form-data"方式提交
boolean isMulti = ServletFileUpload.isMultipartContent(request);
if (isMulti) {
// 通過FileItemFactory得到文件上傳的對象
FileItemFactory fif = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(fif);
try {
ListFileItem> items = upload.parseRequest(request);
for (FileItem item : items) {
// 判斷是否是普通表單控件,或者是文件上傳表單控件
boolean isForm = item.isFormField();
if (isForm) {// 是普通表單控件
String name = item.getFieldName();
if ("id".equals(name)) {
id = Integer.parseInt(item.getString("utf-8"));
System.out.println(id);
}
if ("sex".equals(name)) {
sex = item.getString("utf-8");
System.out.println(sex);
}
if ("username".equals(name)) {
username = item.getString("utf-8");
System.out.println(username);
}
if ("password".equals(name)) {
password = item.getString("utf-8");
System.out.println(password);
}
if ("birthday".equals(name)) {
String birthdayStr = item.getString("utf-8");
SimpleDateFormat sdf = new SimpleDateFormat(
"yyyy-MM-dd");
try {
birthday = sdf.parse(birthdayStr);
} catch (ParseException e) {
e.printStackTrace();
}
System.out.println(birthday);
}
if ("address".equals(name)) {
address = item.getString("utf-8");
System.out.println(address);
}
if ("picturePath".equals(name)) {
picturePath = item.getString("utf-8");
System.out.println(picturePath);
}
} else {// 是文件上傳表單控件
// 得到文件名 xxx.jpg
String sourceFileName = item.getName();
// 得到文件名的擴展名:.jpg
String extendedName = sourceFileName.substring(
sourceFileName.lastIndexOf("."),
sourceFileName.length());
// 把文件名稱重命名為全球唯一的文件名
String uniqueName = UUID.randomUUID().toString();
saveFileName = uniqueName + extendedName;
// 得到上傳到服務器上的文件路徑
// C:\\apache-tomcat-7.0.47\\webapps\\taobaoServlet4\\upload\\xx.jpg
String uploadFilePath = request.getSession()
.getServletContext().getRealPath("upload/");
File saveFile = new File(uploadFilePath, saveFileName);
// 把保存的文件寫出到服務器硬盤上
try {
item.write(saveFile);
} catch (Exception e) {
e.printStackTrace();
}
}
}
} catch (NumberFormatException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (FileUploadException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
// 2、封裝數(shù)據(jù)
User user = new User(id, username, password, sex, birthday, address,
saveFileName);
// 3、調(diào)用邏輯層API
IUserService iUserService = new UserServiceImpl();
// 4、控制跳轉(zhuǎn)
HttpSession session = request.getSession();
if (iUserService.save(user) > 0) {
System.out.println("添加新用戶成功!");
ListUser> users = new ArrayListUser>();
users = iUserService.listAll();
session.setAttribute("users", users);
response.sendRedirect("UserServlet?action=getPage");
} else {
System.out.println("添加新用戶失??!");
PrintWriter out = response.getWriter();
out.print("script type='text/javascript'>");
out.print("alert('添加新用戶失??!請重試!');");
out.print("/script>");
}
}
修改用戶時注意考慮圖片更改和沒更改這兩種情況,圖片更改時要先獲取原圖片并刪除其在服務器上的圖片,再添加新圖片到服務器;圖片不更改時則無需更新圖片路徑。
// 改
public void update(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
System.out.println("update方法被調(diào)用");
HttpSession session = request.getSession();
// 獲取數(shù)據(jù)
int id = (int)session.getAttribute("id");
String username = null;
String password = null;
String sex = null;
Date birthday = null;
String address = null;
String saveFileName = null;
String picturePath = null;
IUserService iUserService = new UserServiceImpl();
// 得到表單是否以enctype="multipart/form-data"方式提交
boolean isMulti = ServletFileUpload.isMultipartContent(request);
if (isMulti) {
// 通過FileItemFactory得到文件上傳的對象
FileItemFactory fif = new DiskFileItemFactory();
ServletFileUpload upload = new ServletFileUpload(fif);
try {
ListFileItem> items = upload.parseRequest(request);
for (FileItem item : items) {
// 判斷是否是普通表單控件,或者是文件上傳表單控件
boolean isForm = item.isFormField();
if (isForm) {// 是普通表單控件
String name = item.getFieldName();
if ("sex".equals(name)) {
sex = item.getString("utf-8");
System.out.println(sex);
}
if ("username".equals(name)) {
username = item.getString("utf-8");
System.out.println(username);
}
if ("password".equals(name)) {
password = item.getString("utf-8");
System.out.println(password);
}
if ("birthday".equals(name)) {
String birthdayStr = item.getString("utf-8");
SimpleDateFormat sdf = new SimpleDateFormat(
"yyyy-MM-dd");
try {
birthday = sdf.parse(birthdayStr);
} catch (ParseException e) {
e.printStackTrace();
}
System.out.println(birthday);
}
if ("address".equals(name)) {
address = item.getString("utf-8");
System.out.println(address);
}
if ("picturePath".equals(name)) {
picturePath = item.getString("utf-8");
System.out.println(picturePath);
}
} else {// 是文件上傳表單控件
// 得到文件名 xxx.jpg
picturePath = item.getName();
if (picturePath != "") {// 有選擇要上傳的圖片
// 得到文件名的擴展名:.jpg
String extendedName = picturePath.substring(
picturePath.lastIndexOf("."),// 截取從最后一個'.'到字符串結(jié)束的子串。
picturePath.length());
// 把文件名稱重命名為全球唯一的文件名
String uniqueName = UUID.randomUUID().toString();
saveFileName = uniqueName + extendedName;// 拼接路徑名
// 得到上傳到服務器上的文件路徑
// C:\\apache-tomcat-7.0.47\\webapps\\CommonhelloWorldServlet\\upload\\xx.jpg
String uploadFilePath = request.getSession()
.getServletContext().getRealPath("upload/");
File saveFile = new File(uploadFilePath,
saveFileName);
// 把保存的文件寫出到服務器硬盤上
try {
item.write(saveFile);
} catch (Exception e) {
e.printStackTrace();
}
// 3、調(diào)用邏輯層 API
// 根據(jù)id查詢用戶并獲取其之前的圖片
User user = iUserService.getUserById(id);
String oldPic = user.getPicturePath();
String oldPicPath = uploadFilePath + "\\" + oldPic;
File oldPicTodelete = new File(oldPicPath);
oldPicTodelete.delete();// 刪除舊圖片
}
}
}
} catch (NumberFormatException e) {
e.printStackTrace();
} catch (FileUploadException e) {
e.printStackTrace();
}
}
System.out.println(id + "\t" + username + "\t" + password + "\t" + sex
+ "\t" + address + "\t" + picturePath + "\t" + birthday);
// 2、封裝數(shù)據(jù)
User user = new User(id, username, password, sex, birthday, address,
saveFileName);
if (iUserService.update(user) > 0) {
System.out.println("修改數(shù)據(jù)成功!");
ListUser> users = new ArrayListUser>();
users = iUserService.listAll();
session.setAttribute("users", users);
// 4、控制跳轉(zhuǎn)
response.sendRedirect("UserServlet?action=getPage");
} else {
System.out.println("修改數(shù)據(jù)失敗!");
PrintWriter out = response.getWriter();
out.print("script type='text/javascript'>");
out.print("alert('修改數(shù)據(jù)失?。≌堉卦?!');");
out.print("/script>");
}
}
刪除的話就比較簡單了,直接獲取原圖片路徑并刪除,則原圖片在服務器上被刪除。
以上就是本文的全部內(nèi)容,希望對大家的學習有所幫助,也希望大家多多支持腳本之家。
您可能感興趣的文章:- js實現(xiàn)圖片上傳到服務器和回顯
- Node.js HTTP服務器中的文件、圖片上傳的方法
- NodeJs實現(xiàn)簡易WEB上傳下載服務器
- 詳解Node.js一行命令上傳本地文件到服務器
- js實現(xiàn)圖片粘貼上傳到服務器并展示的實例
- 基于HTML5+js+Java實現(xiàn)單文件文件上傳到服務器功能
- 利用nodejs監(jiān)控文件變化并使用sftp上傳到服務器
- NodeJS與HTML5相結(jié)合實現(xiàn)拖拽多個文件上傳到服務器的實現(xiàn)方法
- Ajax上傳實現(xiàn)根據(jù)服務器端返回數(shù)據(jù)進行js處理的方法
- js實現(xiàn)上傳圖片到服務器