本文實(shí)例講述了Laravel框架實(shí)現(xiàn)多個視圖共享相同數(shù)據(jù)的方法。分享給大家供大家參考,具體如下:
最近在用Laravel寫一個cms,還沒有完成,但是也遇到了許多難點(diǎn),比如cms后臺每個視圖都要展示相同的導(dǎo)航菜單數(shù)據(jù)。
環(huán)境:
PHP 7.1
Apache 2.4
MySQL 5.7
Laravel 5.4
假設(shè)使用傳統(tǒng)的方法,應(yīng)該是在每個控制器中都調(diào)用數(shù)據(jù),然后把數(shù)據(jù)都塞給視圖。
新建一個BaseController,然后讓BaseController去獲取數(shù)據(jù),然后在每個控制器都繼承BaseController,最后將數(shù)據(jù)塞到視圖中。
基類
class BaseController{
protected $menu = null;//菜單數(shù)據(jù)
public function __construct(){
$this->getMenu();//獲取導(dǎo)航菜單
}
public function getMenu(){
$this->menu = DB::table('menu')->get();
}
}
A控制器
class AController extends BaseController{
public function index(){
return view('admin.index',['menu'=>$this->menu,'user'=>$user]);
}
}
缺點(diǎn):在每個控制器中都需要重新設(shè)置相同的模板的數(shù)據(jù)(menu)
使用Laravel中的View Composers來解決這個問題
1、在App\Providers下創(chuàng)建一個ComposerServiceProvider類
?php
namespace App\Providers;
use Illuminate\Support\Facades\View;
use Illuminate\Support\ServiceProvider;
class ComposerServiceProvider extends ServiceProvider {
/**
* Register bindings in the container.
*
* @return void
*/
public function boot() {
// 基于類的view composer
View::composer(
'admin.common.*', 'App\Http\ViewComposers\AdminComposer'
);
}
/**
* Register the service provider.
*
* @return void
*/
public function register() {
//
}
}
在boot方法中定義要監(jiān)聽的視圖,還可以使用通配符,這里我寫的是admin.common.*,如果admin.common.* 下的視圖被渲染的話將會調(diào)用App\Http\ViewComposers\AdminComposer@composer 方法
2、注冊ComposerServiceProvider
在config/app.php文件下的providers數(shù)組中進(jìn)行注冊
App\Providers\ComposerServiceProvider::class,
3、創(chuàng)建AdminComposer類
Laravel推薦把view composer類放在app\Http\ViewComposers目錄下,這個目錄一開始是沒有的,需要新建
?php
namespace App\Http\ViewComposers;
use App\Libs\CommonUtils;
use Illuminate\Http\Request;
use Illuminate\View\View;
class AdminComposer {
private $data = null;//CommonUtils對象
public function __construct(Request $request) {
$this->data = new CommonUtils($request);//新建一個CommonUtils對象
}
public function compose(View $view) {
$view->with([
'admin' => $this->data->admin,
'mbx' => $this->data->mbx,
'menu' => $this->data->menu,
'msg' => $this->data->msg
]);//填充數(shù)據(jù)
}
}
在這里我在構(gòu)造方法中創(chuàng)建了一個對象,這個對象中包含著數(shù)據(jù)
5、CommonUtils文件
?php
/**
* Created by PhpStorm.
* User: Administrator
* Date: 2017/4/20 0020
* Time: 19:49
*/
namespace App\Libs;
use App\Admin;
use App\Perm;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\DB;
class CommonUtils {
public $admin = null;//管理員對象
public $menu = null;//菜單對象
public $mbx = null;//面包屑對象
public $msg = null;//消息對象
/**
* 構(gòu)造函數(shù)
*/
public function __construct(Request $request) {
$this->init($request);
}
/**
* 初始化函數(shù)
*/
private function init(Request $request) {
$this->getAdmin($request);
$this->getMsg();
$this->getMenu($request);
$this->getMbx($request);
}
/**
* 獲取管理員數(shù)據(jù)
*/
private function getAdmin() {
$this->admin = session('admin');
}
/**
* 獲取后臺菜單數(shù)據(jù)
*/
private function getMenu(Request $request) {
$menu = DB::table('menu')->where('parentid', 0)->orderBy('sort')->get();
$router = $request->getPathInfo();
$perm = new Perm();
$mbx = $perm->getMbx($router);
foreach ($menu as $k => $m) {
$m->active = '';
//讀取子菜單
$childMenu = DB::table('menu')->where('parentid', $m->id)->orderBy('sort')->get();
if (count($childMenu) > 0) {
foreach($childMenu as $v){
$v->active = '';
if($mbx[0]->router == $v->router){
$v->active = 'active';
$m->active = 'active';
}
}
$m->childMenu = $childMenu;
} else {
$m->childMenu = null;
}
}
$this->menu = $menu;
}
/**
* 獲取面包屑
*/
private function getMbx(Request $request) {
$router = $request->getPathInfo();
$perm = new Perm();
$mbx = $perm->getMbx($router);
$this->mbx = $mbx;
}
/**
* 獲取未讀消息
*/
private function getMsg() {
$adminModel = new Admin();
$toId = $this->admin->id;
$this->msg = $adminModel->getUnReadMsg($toId);
}
}
在這里面分別獲取了管理員、菜單、面包屑、消息數(shù)據(jù),這些數(shù)據(jù)都是每個后臺頁面都要使用到的。
注意:這里我將類定義成了CommonUtils,感覺名字取得不好,CommonUtils是存放在App\Libs下的,這個Libs文件夾是我新建的,用于存放工具類的。如果需要給App\Libs文件夾添加自動加載,需要在composer.json文件里做如下修改。
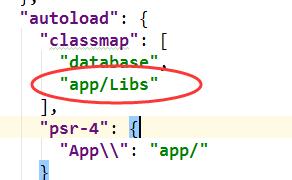
更多關(guān)于Laravel相關(guān)內(nèi)容感興趣的讀者可查看本站專題:《Laravel框架入門與進(jìn)階教程》、《php優(yōu)秀開發(fā)框架總結(jié)》、《php面向?qū)ο蟪绦蛟O(shè)計(jì)入門教程》、《php+mysql數(shù)據(jù)庫操作入門教程》及《php常見數(shù)據(jù)庫操作技巧匯總》
希望本文所述對大家基于Laravel框架的PHP程序設(shè)計(jì)有所幫助。
您可能感興趣的文章:- laravel多視圖共享數(shù)據(jù)實(shí)例代碼