本文實例講述了PHP設(shè)計模式入門之狀態(tài)模式原理與實現(xiàn)方法。分享給大家供大家參考,具體如下:
想必大家都用過自動售賣的自動飲料機吧,塞入硬幣或紙幣,選擇想要的飲料,飲料就會在機器的下方滾出。大家有沒有相關(guān)如果用程序去寫一個飲料機要怎么樣實現(xiàn)呢?
首先我們可以分享一下這部飲料機有幾種狀態(tài)
一、沒有錢的狀態(tài)
二、有錢的狀態(tài)
三、售出的狀態(tài)
四、銷售一空的狀態(tài)
好吧,知道了這些狀態(tài)之后我們開始寫代碼了!
JuiceMachine.php
?php
/**
* 飲料機
* @author ben
*
*/
class JuiceMachine{
/**
* 糖果機一共存在四種狀態(tài):沒錢,有錢,成功售出以及銷售一空
*
* 沒錢的狀態(tài)
* @var INT
*/
const NOMONEY = 0;
/**
* 有錢的狀態(tài)
* @var INT
*/
const HASMONEY = 1;
/**
* 成功售出的狀態(tài)
* @var INT
*/
const SOLD = 2;
/**
* 銷售一空的狀態(tài)
* @var INT
*/
const SOLDOUT = 3;
/**
* 記錄糖果機當前的狀態(tài),初始化狀態(tài)為售空
* @var INT
*/
private $_state = JuiceMachine::SOLDOUT;
/**
* 該變量用于記錄飲料機中飲料的數(shù)量
*/
private $_count;
/**
* 構(gòu)造方法,最主要是用來初始化count和state屬性的
*/
public function __construct($count){
$this->_count = $count;
//當飲料機中的飲料數(shù)量大于零時,將飲料機的狀態(tài)重置為沒有錢的狀態(tài)。
if($this->_count > 0){
$this->_state = JuiceMachine::NOMONEY;
}
}
/**
* 投入硬幣
*/
public function insertCoin(){
if($this->_state == JuiceMachine::HASMONEY ){
echo "you can't insert another coin!br />";
}elseif($this->_state == JuiceMachine::NOMONEY){
echo "you just insert a coinbr />";
$this->_state = JuiceMachine::HASMONEY;
}elseif($this->_state == JuiceMachine::SOLD){
echo "wait a minute, we are giving you a bottle of juicebr />";
}elseif($this->_state == JuiceMachine::SOLDOUT){
echo "you can't insert coin, the machine is already soldoutbr />";
}
}
/**
* 退回硬幣
*/
public function retreatCoin(){
if($this->_state == JuiceMachine::HASMONEY ){
echo "coin return!br />";
$this->_state = JuiceMachine::NOMONEY;
}elseif($this->_state == JuiceMachine::NOMONEY){
echo "you have'nt inserted a coin yetbr />";
}elseif($this->_state == JuiceMachine::SOLD){
echo "sorry, you already clicked the bottonbr />";
}elseif($this->_state == JuiceMachine::SOLDOUT){
echo "you have'nt inserted a coin yetbr />";
}
}
/**
* 點擊飲料對應(yīng)的按鈕
*/
public function clickButton(){
if($this->_state == JuiceMachine::HASMONEY ){
echo "you clicked, we are giving you a bottle of juice...br />";
$this->_state = JuiceMachine::SOLD; //改變飲料機的狀態(tài)為售出模式
$this->dispend();
}elseif($this->_state == JuiceMachine::NOMONEY){
echo "you clicked,but you hav'nt inserted a coin yetbr />";
}elseif($this->_state == JuiceMachine::SOLD){
echo "click twice does'nt get you two bottle of juicebr />";
}elseif($this->_state == JuiceMachine::SOLDOUT){
echo "you clicked, but the machine is already soldoutbr />";
}
}
/**
* 發(fā)放飲料
*/
public function dispend(){
if($this->_state == JuiceMachine::HASMONEY ){
echo "please click the button firstbr />";
}elseif($this->_state == JuiceMachine::NOMONEY){
echo "you need to pay firstbr />";
}elseif($this->_state == JuiceMachine::SOLD){
echo "now you get you juicebr />";
//飲料機中的飲料數(shù)量減一
$this->_count--;
if($this->_count = 0){
echo "opps, runing out of juicebr />";
//如果這時飲料機中沒有飲料了,將飲料機的狀態(tài)重置為銷售一空
$this->_state = JuiceMachine::SOLDOUT;
}else{
//將飲料機的狀態(tài)重置為沒有錢
$this->_state = JuiceMachine::NOMONEY;
}
}elseif($this->_state == JuiceMachine::SOLDOUT){
//其實這種情況不應(yīng)該出現(xiàn)
echo "opps, it appears that we don't have any juice leftbr />";
}
}
}
index.php
?php
require_once 'JuiceMachine.php';
$juiceMachine = new JuiceMachine(1);
$juiceMachine->insertCoin();
$juiceMachine->clickButton();
運行的結(jié)果是:
you just insert a coin
you clicked, we are giving you a bottle of juice...
now you get you juice
opps, runing out of juice
到目前為止我們的程序運行良好,沒有出現(xiàn)什么問題,但是從這些多重的if判斷中你是否嗅到了壞代碼的味道呢?有一天問題終于出現(xiàn)了,老板希望當用戶點擊按鈕時有10%的概率拿到兩瓶飲料,我們需要為飲料機多加一個狀態(tài),這時去修改代碼就成為了一種災(zāi)難,而且很可能會影響到之前的代碼,帶來新的bug,看看狀態(tài)模式如何幫助我們度過難關(guān)吧!
狀態(tài)模式的官方定義是:狀態(tài)模式允許對象在內(nèi)部狀態(tài)改變是改變它的行為,對象看起來好像是修改了它的類
用uml類圖表示如下:
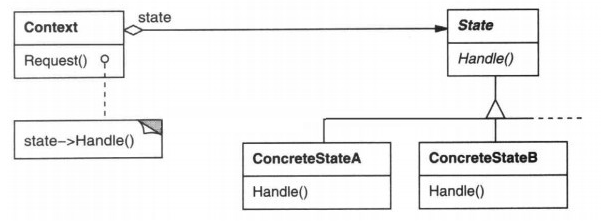
在我們這個項目中的實際類圖如下:
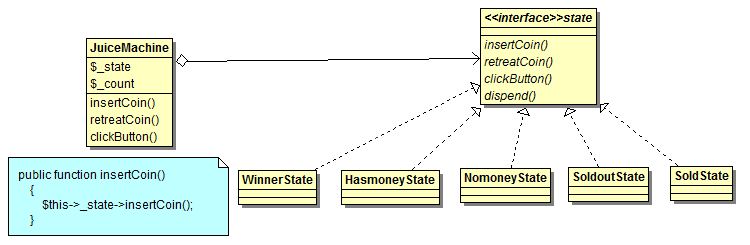
具體實現(xiàn)代碼:
State.php
?php
interface State{
/**
* 插入硬幣
*/
public function insertCoin();
/**
* 回退硬幣
*/
public function retreatCoin();
/**
* 點擊按鈕
*/
public function clickButton();
/**
* 發(fā)放飲料
*/
public function dispend();
}
NomoneyState.php
?php
require_once 'State.php';
class NomoneyState implements State{
/**
* 飲料機的實例
*
* @var object
*/
private $_juiceMachine;
/**
* 構(gòu)造方法,主要用于初始化飲料機實例
*
*/
public function __construct($juiceMachine){
$this->_juiceMachine = $juiceMachine;
}
/* (non-PHPdoc)
* @see State::insertCoin()
*/
public function insertCoin()
{
// TODO Auto-generated method stub
echo "you just insert a coinbr />";
//將飲料機的狀態(tài)切換成有錢的狀態(tài)
$this->_juiceMachine->setState($this->_juiceMachine->getHasmoneyState());
}
/* (non-PHPdoc)
* @see State::retreatCoin()
*/
public function retreatCoin()
{
// TODO Auto-generated method stub
echo "you have'nt inserted a coin yetbr />";
}
/* (non-PHPdoc)
* @see State::clickButton()
*/
public function clickButton()
{
// TODO Auto-generated method stub
echo "you clicked,but you hav'nt inserted a coin yetbr />";
}
/* (non-PHPdoc)
* @see State::dispend()
*/
public function dispend()
{
// TODO Auto-generated method stub
echo "you need to pay firstbr />";
}
}
HasmoneyState.php
?php
require_once 'State.php';
class HasmoneyState implements State
{
/**
* 飲料機的實例
*
* @var object
*/
private $_juiceMachine;
/**
* 構(gòu)造方法,主要用于初始化飲料機實例
*/
public function __construct($juiceMachine)
{
$this->_juiceMachine = $juiceMachine;
}
/*
* (non-PHPdoc) @see State::insertCoin()
*/
public function insertCoin()
{
// TODO Auto-generated method stub
echo "you can't insert another coin!br />";
}
/*
* (non-PHPdoc) @see State::retreatCoin()
*/
public function retreatCoin()
{
// TODO Auto-generated method stub
echo "coin return!br />";
$this->_juiceMachine->setState($this->_juiceMachine->getNomoneyState());
}
/*
* (non-PHPdoc) @see State::clickButton()
*/
public function clickButton()
{
// TODO Auto-generated method stub
echo "you clicked, we are giving you a bottle of juice...br />";
// 改變飲料機的狀態(tài)為售出模式
$rand = mt_rand(0, 0);
// 當隨機數(shù)為0(即1/10的概率)并且飲料機中還有1瓶以上的飲料時
if ($rand == 0 $this->_juiceMachine->getCount() > 1) {
$this->_juiceMachine->setState($this->_juiceMachine->getWinnerState());
} else {
$this->_juiceMachine->setState($this->_juiceMachine->getSoldState());
}
}
/*
* (non-PHPdoc) @see State::dispend()
*/
public function dispend()
{
// TODO Auto-generated method stub
echo "please click the button firstbr />";
}
}
SoldoutState.php
?php
require_once 'State.php';
class SoldoutState implements State{
/**
* 飲料機的實例
*
* @var object
*/
private $_juiceMachine;
/**
* 構(gòu)造方法,主要用于初始化飲料機實例
*
*/
public function __construct($juiceMachine){
$this->_juiceMachine = $juiceMachine;
}
/* (non-PHPdoc)
* @see State::insertCoin()
*/
public function insertCoin()
{
// TODO Auto-generated method stub
echo "you can't insert coin, the machine is already soldoutbr />";
}
/* (non-PHPdoc)
* @see State::retreatCoin()
*/
public function retreatCoin()
{
// TODO Auto-generated method stub
echo "you have'nt inserted a coin yetbr />";
}
/* (non-PHPdoc)
* @see State::clickButton()
*/
public function clickButton()
{
// TODO Auto-generated method stub
echo "you clicked, but the machine is already soldoutbr />";
}
/* (non-PHPdoc)
* @see State::dispend()
*/
public function dispend()
{
// TODO Auto-generated method stub
echo "opps, it appears that we don't have any juice leftbr />";
}
}
SoldState.php
?php
require_once 'State.php';
class SoldState implements State{
/**
* 飲料機的實例
*
* @var object
*/
private $_juiceMachine;
/**
* 構(gòu)造方法,主要用于初始化飲料機實例
*
*/
public function __construct($juiceMachine){
$this->_juiceMachine = $juiceMachine;
}
/* (non-PHPdoc)
* @see State::insertCoin()
*/
public function insertCoin()
{
// TODO Auto-generated method stub
echo "wait a minute, we are giving you a bottle of juicebr />";
}
/* (non-PHPdoc)
* @see State::retreatCoin()
*/
public function retreatCoin()
{
// TODO Auto-generated method stub
echo "sorry, you already clicked the bottonbr />";
}
/* (non-PHPdoc)
* @see State::clickButton()
*/
public function clickButton()
{
// TODO Auto-generated method stub
echo "click twice does'nt get you two bottle of juicebr />";
}
/* (non-PHPdoc)
* @see State::dispend()
*/
public function dispend()
{
$this->_juiceMachine->decJuice();
if($this->_juiceMachine->getCount() = 0){
echo "opps, runing out of juicebr />";
//如果這時飲料機中沒有飲料了,將飲料機的狀態(tài)重置為銷售一空
$this->_juiceMachine->setState($this->_juiceMachine->getSoldoutState());
}else{
//將飲料機的狀態(tài)重置為沒有錢
$this->_juiceMachine->setState($this->_juiceMachine->getNomoneyState());
}
}
}
WinnerState.php
?php
require_once 'State.php';
class WinnerState implements State
{
/**
* 飲料機的實例
*
* @var object
*/
private $_juiceMachine;
/**
* 構(gòu)造方法,主要用于初始化飲料機實例
*/
public function __construct($juiceMachine)
{
$this->_juiceMachine = $juiceMachine;
}
/*
* (non-PHPdoc) @see State::insertCoin()
*/
public function insertCoin()
{
// TODO Auto-generated method stub
echo "wait a minute, we are giving you a bottle of juicebr />";
}
/*
* (non-PHPdoc) @see State::retreatCoin()
*/
public function retreatCoin()
{
// TODO Auto-generated method stub
echo "sorry, you already clicked the bottonbr />";
}
/*
* (non-PHPdoc) @see State::clickButton()
*/
public function clickButton()
{
// TODO Auto-generated method stub
echo "click twice does'nt get you two bottle of juicebr />";
}
/*
* (non-PHPdoc) @see State::dispend()
*/
public function dispend()
{
echo "you are a winner! you get two bottle of juice!br />";
$this->_juiceMachine->decJuice();
if ($this->_juiceMachine->getCount() > 0) {
$this->_juiceMachine->decJuice();
if ($this->_juiceMachine->getCount() = 0) {
echo "opps, runing out of juicebr />";
// 如果這時飲料機中沒有飲料了,將飲料機的狀態(tài)重置為銷售一空
$this->_juiceMachine->setState($this->_juiceMachine->getSoldoutState());
} else {
// 將飲料機的狀態(tài)重置為沒有錢
$this->_juiceMachine->setState($this->_juiceMachine->getSoldoutState());
}
} else {
echo "opps, runing out of juicebr />";
// 如果這時飲料機中沒有飲料了,將飲料機的狀態(tài)重置為銷售一空
$this->_juiceMachine->setState($this->_juiceMachine->getSoldoutState());
}
}
}
JuiceMachine.php
?php
require_once './state/NomoneyState.php';
require_once './state/HasmoneyState.php';
require_once './state/SoldState.php';
require_once './state/SoldoutState.php';
require_once './state/WinnerState.php';
class JuiceMachine
{
/**
* 記錄糖果機當前的狀態(tài),初始化狀態(tài)為售空
*
* @var object
*/
private $_state;
/**
* 該變量用于記錄飲料機中飲料的數(shù)量
*/
private $_count;
/**
* 構(gòu)造方法,最主要是用來初始化count和state屬性的
*/
public function __construct($count)
{
$this->_state = new SoldoutState($this);
$this->_count = $count;
// 當飲料機中的飲料數(shù)量大于零時,將飲料機的狀態(tài)重置為沒有錢的狀態(tài)。
if ($this->_count > 0) {
$this->_state = new NomoneyState($this);
}
}
/*
* (non-PHPdoc) @see State::insertCoin()
*/
public function insertCoin()
{
// TODO Auto-generated method stub
$this->_state->insertCoin();
}
/*
* (non-PHPdoc) @see State::retreatCoin()
*/
public function retreatCoin()
{
// TODO Auto-generated method stub
$this->_state->retreatCoin();
}
/*
* (non-PHPdoc) @see State::clickButton()
*/
public function clickButton()
{
$this->_state->clickButton();
//其實發(fā)放糖果是在用戶點擊完按鈕后機器內(nèi)部進行的所有沒有必要再寫一個dispend方法
$this->_state->dispend();
}
/**
* 設(shè)置糖果機的狀態(tài)
*
* @param State $state
*/
public function setState(State $state)
{
$this->_state = $state;
}
/**
* 獲取沒有錢的狀態(tài)
*/
public function getNomoneyState(){
return new NomoneyState($this);
}
/**
* 獲取有錢的狀態(tài)
*/
public function getHasmoneyState(){
return new HasmoneyState($this);
}
/**
* 獲取售出的狀態(tài)
*/
public function getSoldState(){
return new SoldState($this);
}
/**
* 獲取銷售一空的狀態(tài)
*/
public function getSoldoutState(){
return new SoldoutState($this);
}
/**
* 獲取幸運者的狀態(tài)
*/
public function getWinnerState(){
return new WinnerState($this);
}
/**
* 獲取飲料機中飲料的數(shù)量
*/
public function getCount(){
return $this->_count;
}
/**
* 將飲料數(shù)量減一
*/
public function decJuice(){
echo "now you get you juicebr />";
//飲料機中的飲料數(shù)量減一
$this->_count--;
}
}
index.php
?php
require_once 'JuiceMachine.php';
$juiceMachine = new JuiceMachine(2);
$juiceMachine->insertCoin();
$juiceMachine->clickButton();
更多關(guān)于PHP相關(guān)內(nèi)容感興趣的讀者可查看本站專題:《php面向?qū)ο蟪绦蛟O(shè)計入門教程》、《PHP數(shù)組(Array)操作技巧大全》、《PHP基本語法入門教程》、《PHP運算與運算符用法總結(jié)》、《php字符串(string)用法總結(jié)》、《php+mysql數(shù)據(jù)庫操作入門教程》及《php常見數(shù)據(jù)庫操作技巧匯總》
希望本文所述對大家PHP程序設(shè)計有所幫助。
您可能感興趣的文章:- PHP設(shè)計模式之原型模式示例詳解
- PHP設(shè)計模式之命令模式示例詳解
- PHP設(shè)計模式(四)原型模式Prototype實例詳解【創(chuàng)建型】
- PHP設(shè)計模式(三)建造者模式Builder實例詳解【創(chuàng)建型】
- PHP設(shè)計模式(一)工廠模式Factory實例詳解【創(chuàng)建型】
- PHP設(shè)計模式概論【概念、分類、原則等】
- PHP設(shè)計模式之 策略模式Strategy詳解【對象行為型】
- PHP設(shè)計模式入門之迭代器模式原理與實現(xiàn)方法分析
- PHP設(shè)計模式之迭代器模式Iterator實例分析【對象行為型】
- php設(shè)計模式之適配器模式實例分析【星際爭霸游戲案例】
- php設(shè)計模式之迭代器模式實例分析【星際爭霸游戲案例】
- 詳解PHP八大設(shè)計模式